Monads: Beyond Simple Analogies—Reflections on Functional Programming Paradigms
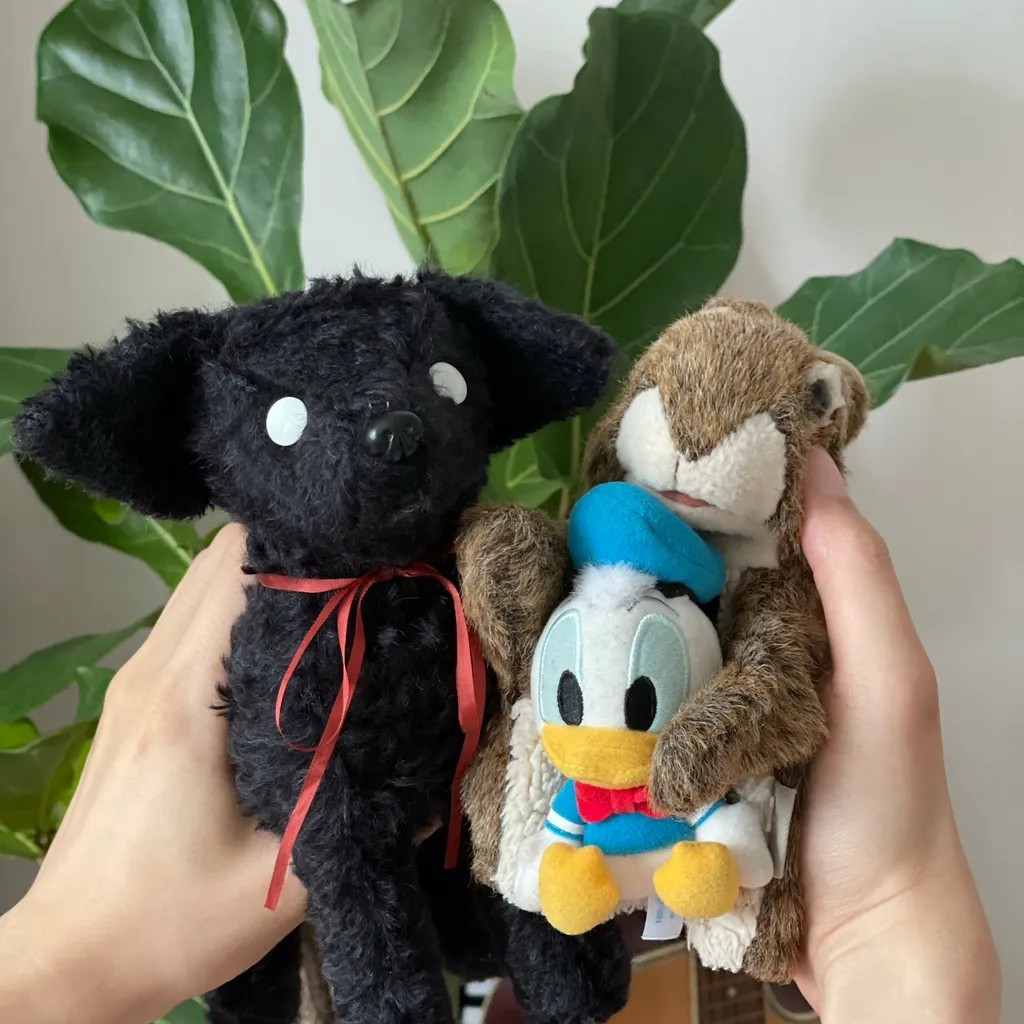
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
While exploring functional programming languages, I've been reflecting on how different communities approach similar concepts. One pattern that seems particularly fascinating is how Haskell and OCaml communities differ in their embrace of monads as an abstraction tool.
The Elegant Power of Monads in Haskell
It's common to hear monads explained through analogies to concepts like JavaScript's Promise
or jQuery chains. While these comparisons provide an entry point, they might miss what makes monads truly beautiful and powerful in Haskell's ecosystem.
The real strength appears to lie in the Monad
typeclass itself. This elegant abstraction allows for creating generic functions and types that work with any type that shares the monad property. This seems to offer a profound unification of concepts that might initially appear unrelated:
- You can write code once that works across many contexts (
Maybe
,[]
,IO
,State
, etc.) - Generic functions like
sequence
,mapM
, and others become available across all monadic types - The same patterns and mental models apply consistently across different computational contexts
For example, a simple conditional function like this works beautifully in any monadic context:
whenM :: Monad m => m Bool -> m () -> m ()
whenM condition action = do
result <- condition
if result then action else return ()
Whether dealing with potentially missing values, asynchronous operations, or state transformations, the same function can be employed without modification. There's something genuinely satisfying about this level of abstraction and reuse.
OCaml's Different Approach
Interestingly, the OCaml community seems less enthusiastic about monads as a primary abstraction tool. This might stem from several factors related to language design:
Structural Differences
OCaml lacks built-in typeclass support, relying instead on its module system and functors. While powerful in its own right, this approach might not make monad abstractions feel as natural or convenient:
(* OCaml monad implementation requires more boilerplate *)
module type MONAD = sig
type 'a t
val return : 'a -> 'a t
val bind : 'a t -> ('a -> 'b t) -> 'b t
end
module OptionMonad : MONAD with type 'a t = 'a option = struct
type 'a t = 'a option
let return x = Some x
let bind m f = match m with
| None -> None
| Some x -> f x
end
OCaml also doesn't offer syntactic sugar like Haskell's do
notation, which makes monadic code in Haskell considerably more readable and expressive:
-- Haskell's elegant do notation
userInfo = do
name <- getLine
age <- readLn
return (name, age)
Compared to the more verbose OCaml equivalent:
let user_info =
get_line >>= fun name ->
read_ln >>= fun age ->
return (name, age)
The readability difference becomes even more pronounced in more complex monadic operations.
Philosophical Differences
Beyond syntax, the languages differ in their fundamental approach to effects:
- Haskell is purely functional, making monads essential for managing effects in a principled way
- OCaml permits direct side effects, often making monadic abstractions optional
This allows OCaml programmers to write more direct code when appropriate:
(* Direct style in OCaml *)
let get_user_info () =
print_string "Name: ";
let name = read_line () in
print_string "Age: ";
let age = int_of_string (read_line ()) in
(name, age)
OCaml's approach might favor pragmatism and directness in many cases, with programmers often preferring:
- Direct use of
option
andresult
types - Module-level abstractions through functors
- Continuation-passing style when needed
While this directness can be beneficial for immediate readability, it might come at the cost of some of the elegant uniformity that Haskell's monadic approach provides.
Reflections on Language Design
These differences highlight how programming language design shapes the idioms and patterns that emerge within their communities. Neither approach is objectively superior—they represent different philosophies about abstraction, explicitness, and the role of the type system.
Haskell's approach encourages a high level of abstraction and consistency across different computational contexts, which can feel particularly satisfying when working with complex, interconnected systems. There's something intellectually pleasing about solving a problem once and having that solution generalize across many contexts.
OCaml often favors more direct solutions that might be easier to reason about locally, though potentially at the cost of less uniformity across the codebase. This approach has its own virtues, particularly for systems where immediate comprehensibility is paramount.
After working with both paradigms, I find myself drawn to the consistent abstractions that Haskell's approach provides, while still appreciating the pragmatic clarity that OCaml can offer in certain situations. The typeclasses and syntactic support in Haskell seem to unlock a particularly elegant way of structuring code that, while perhaps requiring a steeper initial learning curve, offers a uniquely satisfying programming experience.
What patterns have you noticed in how different programming language communities approach similar problems? And have you found yourself drawn to the elegant abstractions of Haskell or the pragmatic approach of OCaml?