클플 뭐 서비스 낼때마다 리전 맨날 Earth 요따구로 해두니까 우주진출 뒤의 클플을 상상하게 됨
XiNiHa
@xiniha@hackers.pub · 79 following · 88 followers
GitHub
- @XiNiHa
Bluesky
- @xiniha.dev
Twitter
- @xiniha_1e88df
XiNiHa shared the below article:
How to pass the invisible
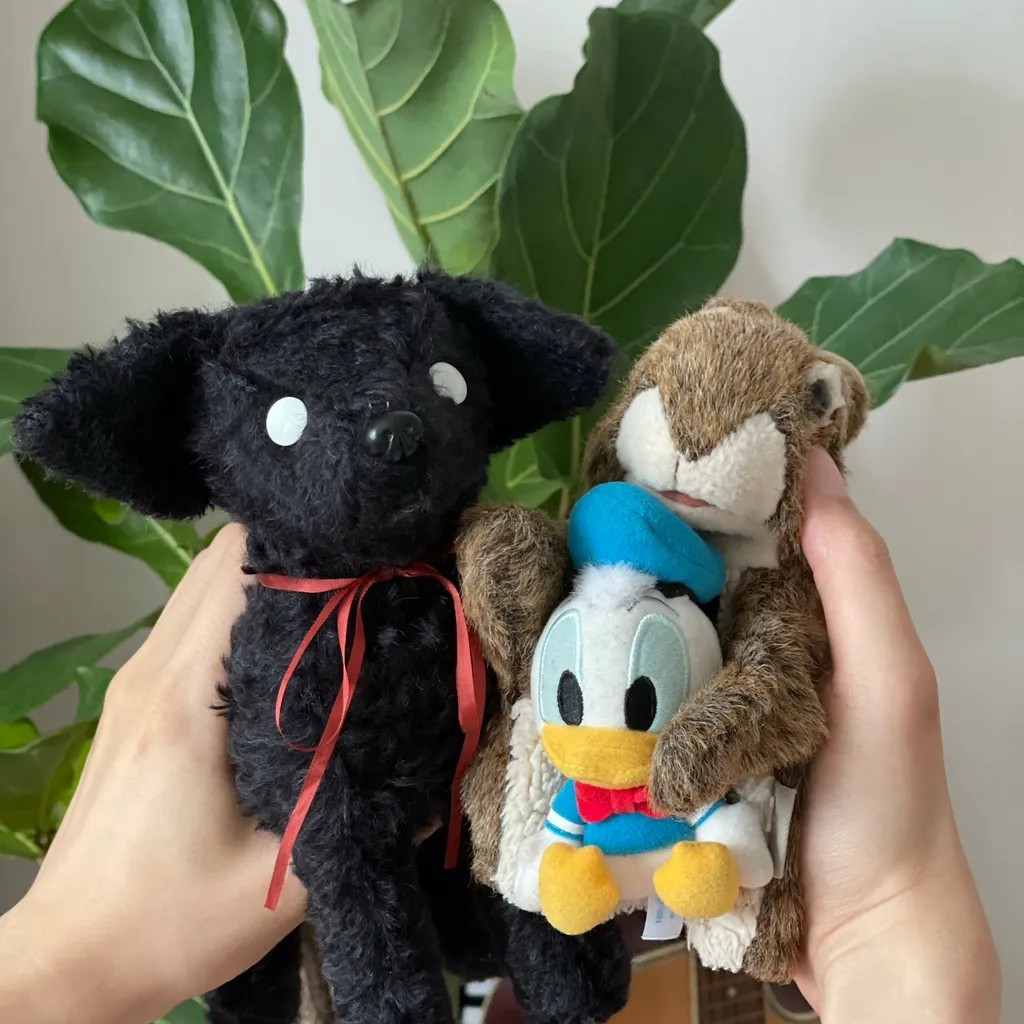
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
This post explores the enduring challenge in software programming of how to pass invisible contextual information, such as loggers or request contexts, through applications without cumbersome explicit parameter passing. It examines various approaches throughout history, including dynamic scoping, aspect-oriented programming (AOP), context variables, monads, and effect systems. Each method offers a unique solution, from the simplicity of dynamic scoping in early Lisp to the modularity of AOP and the type-safe encoding of effects in modern functional programming. The post highlights the trade-offs of each approach, such as the unpredictability of dynamic scoping or the complexity of monad transformers. It also touches on how context variables are used in modern asynchronous and parallel programming, as well as in UI frameworks like React. The author concludes by noting that the art of passing the invisible is an eternal theme in software programming, and this post provides valuable insights into the evolution and future directions of this critical aspect of software architecture.
Read more →Phew! That's it for the merge train: this ended up being a fuller day than I was hoping for. Don't stay up until 2 am kids. At least, not if you're Old™.
Anyways, I'm feeling really pleased about the progress on a lot of controversial features today, between the merge train and the meeting!
#bevy 0.17 is shaping up to be a really exciting release: hot patching, widgets, observers, tilemaps, light textures, transform overhaul... :) Community-driven open source is really wild like that: folks just come along and upset all your plans. Mostly for the better even!
회사에서 AI 도입 드라이브가 강한데 솔직히 하나만 했으면 좋겠다는 생각이 든다. 기존의 방식으로 일하는 것을 그만두든지 AI를 신경쓰지 말든지. 두개를 애매하게 다 하려니까 가랑이가 찢어진다.
실제로 Hackers' Pub은 Mac mini M4 깡통에서 돌아가는데, 아직 Asahi Linux가 M4를 지원 안 해서 macOS 안에 컨테이너 띄워서 돌리고 있어요. Asahi Linux가 M4 지원할 때까지 숨 참고 기다리는 중입니다…
XiNiHa shared the below article:
If you're building a JavaScript library and need logging, you'll probably love LogTape
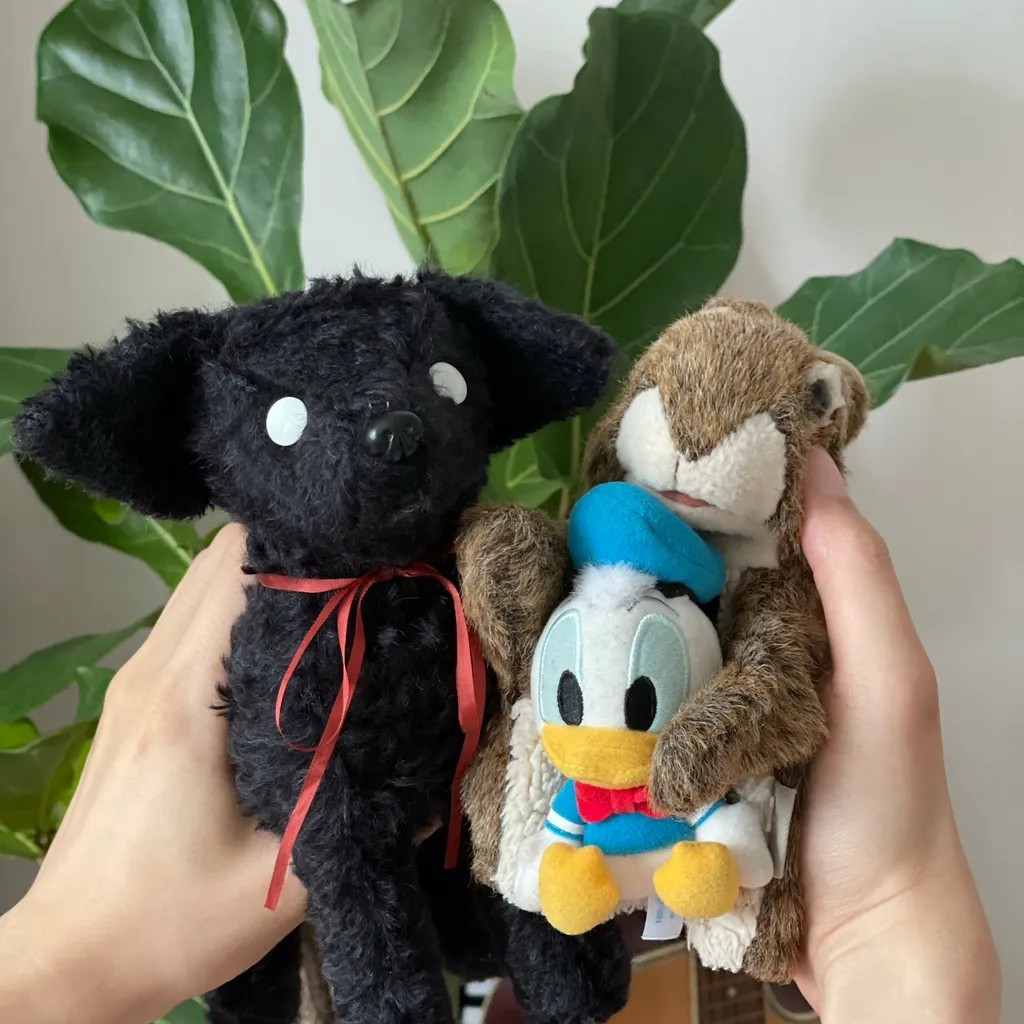
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
LogTape offers a novel approach to logging in JavaScript libraries, designed to provide diagnostic capabilities without imposing choices on users. Unlike traditional methods such as using debug packages or custom logging systems, LogTape operates on a "library-first design" where logging is transparent and only activated when configured. This eliminates the fragmentation problem of managing multiple logging systems across different libraries. With zero dependencies and support for both ESM and CommonJS, LogTape ensures minimal impact on users' projects, avoiding dependency conflicts and enabling tree shaking. Its universal runtime support and efficient performance make it suitable for various environments. By using a hierarchical category system, LogTape prevents namespace collisions, offering a seamless developer experience with TypeScript support and structured logging patterns. LogTape provides adapters for popular logging libraries like Winston and Pino, bridging the transition for users invested in other systems. Ultimately, LogTape offers a way to enhance library capabilities while respecting users' preferences and existing choices, making it a valuable consideration for library authors.
Read more →XiNiHa shared the below article:
Announcing LogTape 1.0.0
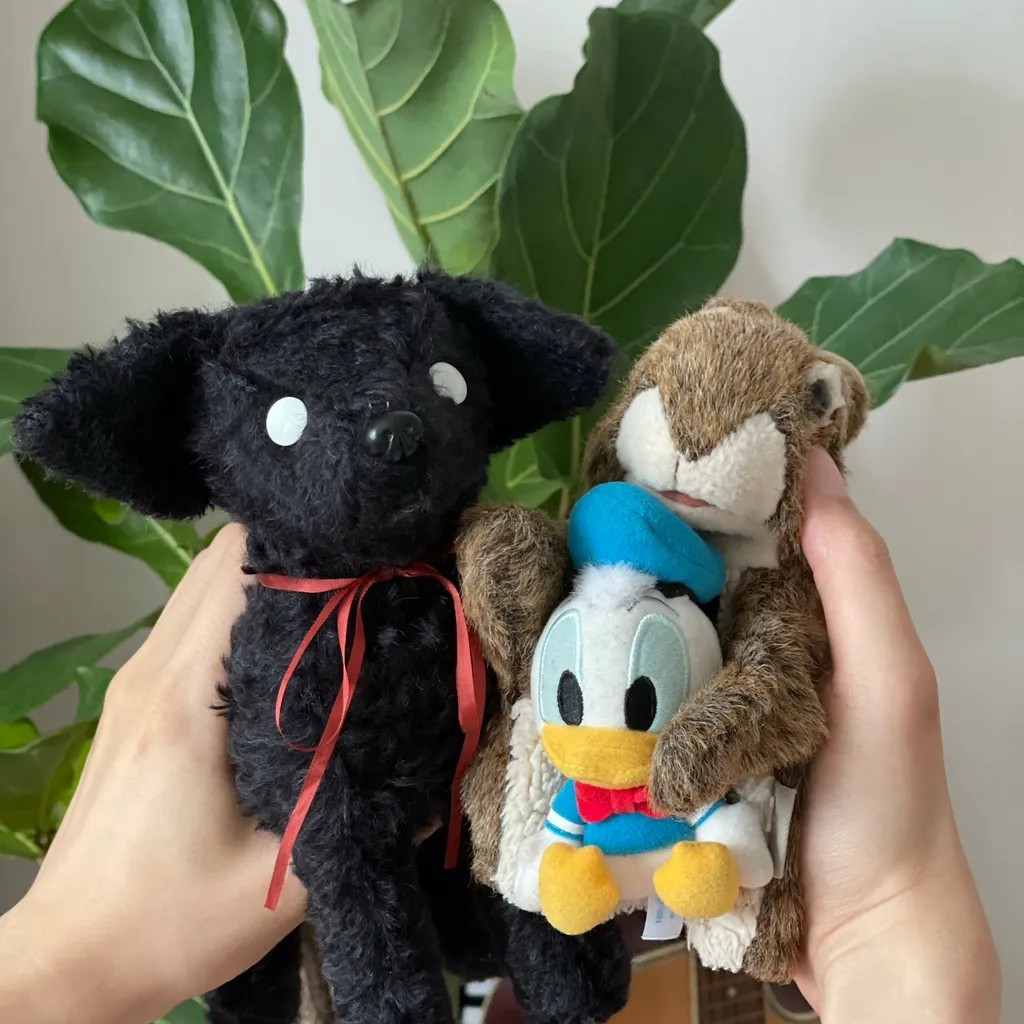
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
LogTape 1.0.0 has been released, marking a significant milestone for this zero-dependency logging library designed for the modern JavaScript ecosystem. This release emphasizes API stability and introduces high-performance features such as non-blocking sinks for console, stream, and file logging, along with the `fromAsyncSink()` function for integrating asynchronous logging operations. New sink integrations include packages for AWS CloudWatch Logs and Windows Event Log, enhancing LogTape's versatility. The update also brings a visually appealing console logging experience with the `@logtape/pretty` package, and seamless integration with existing Winston or Pino setups through adapter packages. Key developer experience enhancements include programmatic access to log levels and improved browser compatibility. LogTape 1.0.0 streamlines logging infrastructure with a comprehensive package ecosystem, offering specialized packages for various logging needs. This release provides a stable and mature logging solution, making it easier to manage and optimize logging in JavaScript applications.
Read more →LogTape 1.0.0 출시 발표
LogTape 1.0.0이 출시되었습니다. 이 로깅 라이브러리는 현대적인 JavaScript 생태계를 위해 설계되었으며, 의존성이 없고 Node.js, Deno, Bun, 브라우저, 엣지 함수 등 다양한 런타임을 지원합니다. LogTape는 라이브러리 우선 설계를 통해 라이브러리 작성자가 사용자에게 부담을 주지 않고 로깅 기능을 추가할 수 있도록 합니다. 이번 릴리스에서는 고성능 로깅 인프라를 위한 비동기 싱크 옵션, AWS CloudWatch Logs 및 Windows Event Log와의 통합, 그리고 개발 경험을 향상시키는 예쁜 콘솔 로깅 기능이 추가되었습니다. 또한, 기존 Winston 및 Pino 로깅 시스템과의 원활한 통합을 위한 어댑터 패키지를 제공하여 LogTape를 사용하는 라이브러리를 쉽게 통합할 수 있도록 지원합니다. LogTape 1.0.0은 안정성과 성숙도를 제공하며, 다양한 로깅 요구 사항을 충족시키는 포괄적인 패키지 생태계를 구축했습니다.
hackers.pub · Hackers' Pub
Link author: 洪 民憙 (Hong Minhee)@hongminhee@hackers.pub
Nginx의 njs에 QuickJS 엔진 추가되니 기능이나 문법 제약도 거의 없어서 출근해서 해볼 성능 테스트만 제대로 되면 좀 복잡한 로직 필요한 리버스 프록시는 이걸로만 짤거같음. JS모듈들 대충 번들로 만들고 import해서 다 쓸수 있어서...
언어 구현을 하는 데에 최고의 언어는 뭘까... 예전엔 OCaml이라고 생각했었고 요즘은 Scala가 최고라고 생각하고 있기는 한데, 더 나은 대안 언어는 없을까? 탈JVM이 하고 싶다 (그렇다고 Scala Native는 아닙니다 진짜...)
@xiniha Pothos is 최고의 GraphQL 서버? 다른걸 안써봐서 모릅니다.
@bglbgl gwyng 그거는 근데 진짜 맞는 것 같긴 해요
근데 진짜 백엔드 개발하는 데에 TS만한 언어가 없는 것 같기도 함
LogTape 0.12.0을 불과 일주일 전에 릴리스했는데, 일주일 만에 또 LogTape 1.0.0 릴리스하기가 좀 뻘쭘하네… 삘 받아서 요 며칠 동안 LogTape 작업만 했더니만.
Zed는 아직 쓰지도 않는데도 요즘 보기드문 장인정신이 느껴지는 소프트웨어라 호감이 간다. 지금 쓰고있는 Windsurf는 VS Code 포크떠서 AI 채팅만 추가했는데 하루에 3번 터져서 에디터를 재시작해야한다(AI 채팅 패널이 터지는거라 VS Code가 아닌 Windsurf 문제로 보임). 5조 투자받은건 어따썼니??
LogTape 다음 버전부터는 널리 쓰이는 로깅 라이브러리인 Pino와 winston에 대한 어댑터를 함께 제공할 예정입니다.
With this latest PR, inference for `noFloatingPromises` is pushed to over 80% success rate in Vercel's repositories: https://github.com/biomejs/biome/pull/6404
While I targeted specific patterns used by their own `swr` library, all the fixes are generically applicable, meaning other code benefits too.
Do you want to use Biome's `noFloatingPromises`, but need support for some library that is important to you? Reach out to us and we can make it happen: https://biomejs.dev/enterprise/
🚀MoonBit Beta is here!
After 2 years of fast iteration, MoonBit enters its stable phase with regard to language syntax!
✨Built-in async
🛠 Fast tooling and IDE-aware error handling
With Biome v2 reaching ~75% accuracy for the `noFloatingPromises` rule, one might wonder, how?
* First of all keep in mind this is measured on a single (very large) repository. Yours might fare differently depending on libraries/patterns/etc..
* What you're seeing is a successful application of the 80/20 rule, which says that by doing 20% of the work, you can reach 80% of the results. So far we're only scratching the surface of #TypeScript, but for most applications, that's all we need.
My Vercel contract is almost coming to an end, but I'm extremely happy that we managed to get to about 75% success rate for `noFloatingPromises` in our v2 release: https://biomejs.dev/blog/biome-v2/
The success rate is even slightly higher already in the `next` branch, and I still have 2 weeks to go. And just to highlight: This is merely the start.
I'll be looking for new means of funding to pursue this work, and thankfully Biome donations are also making a significant difference: https://opencollective.com/biome
오버엔지니어링을 자제해야 하지만 이게 또 일하면서 보람을 느낄 수 있는 방법 중 하나라 타협점을 찾아야겠음
요즘 '이건 잘못된 코드지만 당분간 고칠일이 없기를 기도하자'고 하며 넘어간 코드들에서 나온 버그들을 계속 고치는 중이다.
과거의 나의 정확한 안목에 뿌듯해하며 동시에 피눈물을 흘리고 있다.
mmap을 백엔드로 Vec 같은 인터페이스를 쓸수 있는 프로젝트, memvec 입니다. AI의 힘으로 문서를 보충하여 0.2.0 을 릴리즈했습니다. https://crates.io/crates/memvec
@bglbgl gwyng 손발이 튼튼하면 머리가 고생할 일이 없습니다. 두 벌 구현하면 됩니다! 💨 (LogTape에서 정말로 그렇게 했어요…)
@hongminhee洪 民憙 (Hong Minhee)
@bglbgl gwyng Quansync 안 쓰신 이유가 있으신가요? 👀
어제부터 Jujutsu라는 버전 관리 시스템을 써보고 있습니다. git의 branch는 연속적인 단일 작업을 표현하는 느낌이 강하게 드는데 사실 그저 어느 commit을 가리키는 포인터일 뿐이라는 걸 느끼게 해주네요. Jujutsu에서는 같은 커밋에서 다음 커밋을 여러 개 만들면 그게 브랜치이고, 여러 커밋을 parent로 하는 커밋을 하나 만들면 그게 머지이고, 수정이 다 끝나면 그냥 원하는 브랜치 이름의 포인터를 적절히 옮기면 됩니다. 부분 변경을 커밋 간에 자유롭게 옮길 수 있는 것까지 합치면 재미있는 사용 방법이 많이 있을 것 같습니다. 특히 megamerge workflow를 쓰면 git 쓰다가 생겼던 "지금 하는 작업을 끝내야 다음 변경사항을 작업"하는 강박이 해소될 것 같아 기대가 많이 됩니다.
XiNiHa shared the below article:
LogTape 0.12.0 Release Notes
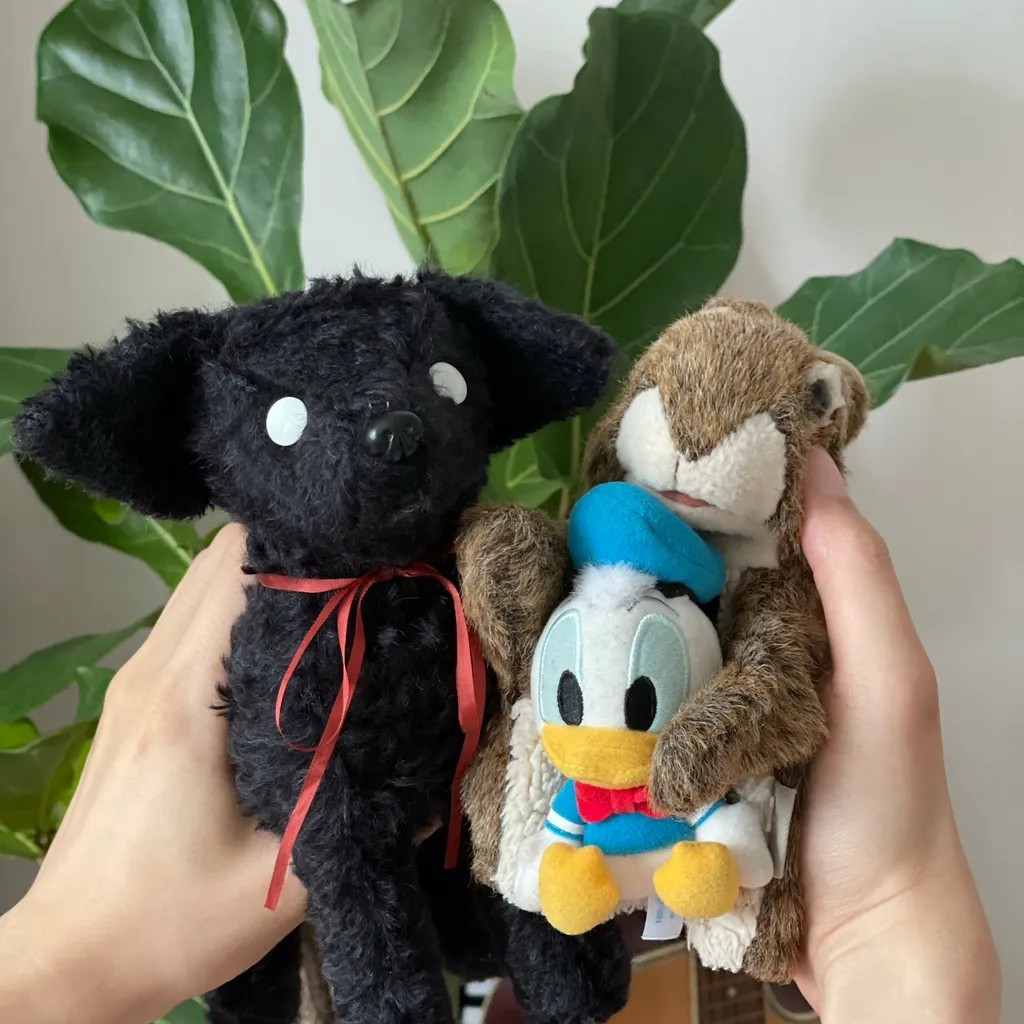
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
LogTape, a zero-dependency logging library for JavaScript and TypeScript, has released version 0.12.0 with several enhancements. The update introduces a new `trace` log level for more granular debugging and improves file sink performance through configurable buffering. A significant addition is the `@logtape/syslog` package, enabling log message transmission to syslog servers using RFC 5424. The update also includes `Logger.warning()` as an alias for `Logger.warn()` for consistency. Furthermore, all LogTape packages now share unified versioning for better compatibility. The build infrastructure has been migrated from `dnt` to `tsdown`, enhancing compatibility with modern JavaScript toolchains and improving build times. This release optimizes logging capabilities and ensures smoother integration with various JavaScript runtimes.
Read more →@xiniha 아하, 아직 하려면 꽤 남았군요?
@hongminhee洪 民憙 (Hong Minhee) 그렇습니다.... 사실 비행기 한 번만 타려고 독일 가는 스케줄도 컨퍼런스 맞춰서 잡았습니다 😂
@xiniha 오… 몇 개 추천해 주세요.
@hongminhee洪 民憙 (Hong Minhee) 아직 스케줄만 나오고 발표가 진행된 건 아니긴 한데 😅 여기에 현재 기준 스케줄이 있습니다
이번 GraphQLConf 발표들도 재밌는 것들 잔뜩이구만
#자기소개 를 해볼까요.
@ranolpRanol☆P 와 동일인입니다...만 해당 계정은 근시일 내에 살릴 계획이 없습니다.
@ranolp 계정은 프로그래밍 언어론/해커스펍 사용기 위주 계정입니다.
- 다시 말하자면 그 외 일상적인 내용은 트위터에서 이야기한다는 뜻입니다...
- TypeScript와 얼추 호환되면서 제정신인 타입 추론 규칙을 가진 언어를 만들려고 타입 이론을 공부하고 있습니다.
- 좀 많이 전에는 Bidirectional Typing (J. Dunfield, N. Krishnaswami)을 읽었었고,
- 독일에 있는 튀빙겐 대학 내에서 연구하는 대수적 효과 언어 Effekt도 간단히 살펴보았었습니다.
- 최근에는 힌들리-밀너-다마스 타입 추론 위에 얹은 부타입 확장을 살펴보고 있습니다.
- 캠브릿지 대학 연구인 MLsub (S. Dolan and A. Mycroft)...
- 을 단순화한 Simple-sub (L. Parreaux)을 시작으로 MLstruct, Ultimate Conditional Syntax 등 홍콩대 연구를 많이 보고 있습니다
- MLscript가 정말 흥미로운 언어에요 ReScript but more Kotlin처럼 생겼음
- 올해 들어서 An Infinitely Large Napkin으로 군론과 군의 작용, 위상수학과 대수 위상(호모토피만), 그리고 범주론을 배웠습니다.
- 형식적 증명 보조기에도 관심이 많습니다.
- Software Foundation을 통해 Coq (현 Rocq)를 약간 배웠습니다.
- Lean 4도 약간 맛보기를 했습니다.
- 의존 타입/마틴 뢰프 타입(MLTT)/호모토피 타입(HoTT) 등을 배워 간단한 증명 보조기도 만들어보고 싶네요.
- 아마 An Infinitely Large Napkin 스터디가 끝나면 HoTT 스터디를 하지 않을까 싶네요.
이런 면에서 KDL이 아주 훌륭하다고 느꼈던 게 /- 주석이란 게 있다. AST 노드 하나를 주석 처리하는 거.
함수형 언어는 뭐 사실 역사와 전통의 리스프 방언에서 시작해야 하지 않나 (웃음)
XiNiHa shared the below article:
Hackers' Pub의 게시글에 번역 힌트 주기
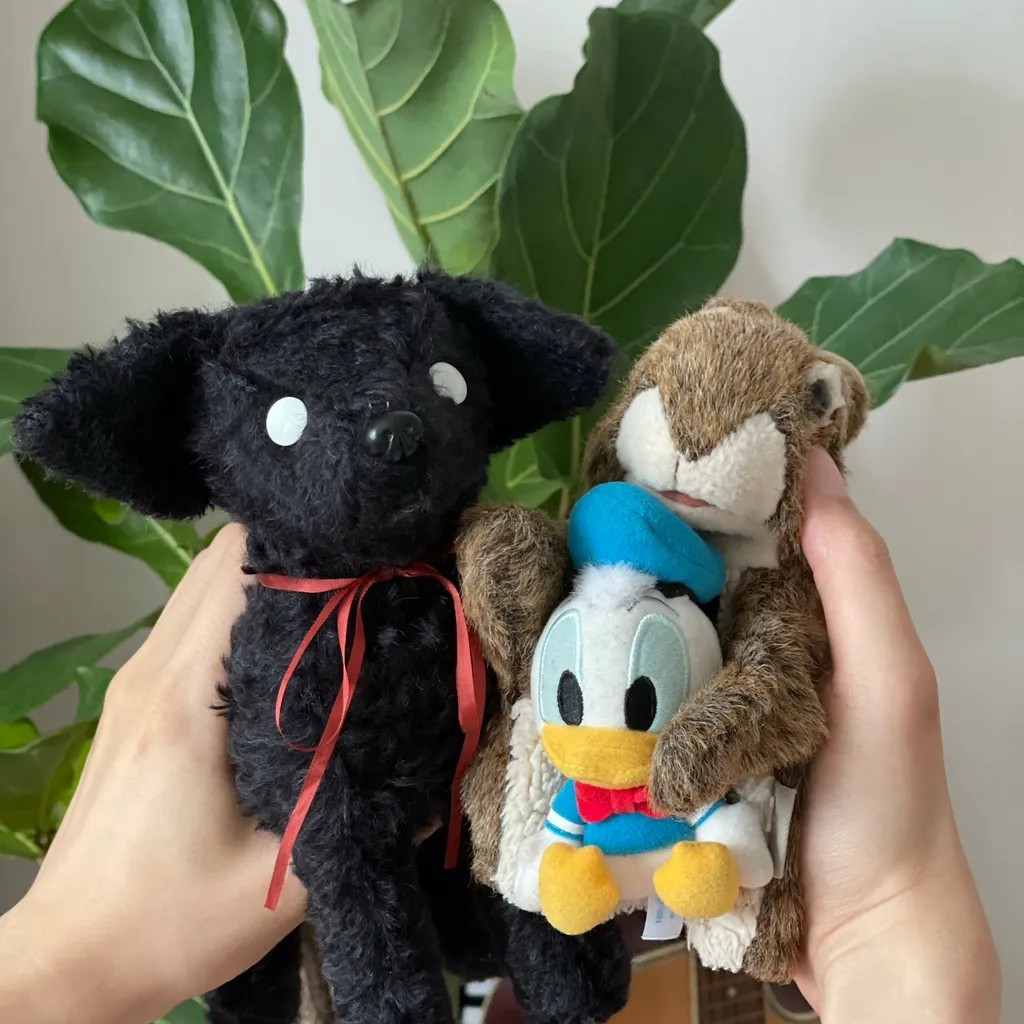
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
Hackers' Pub의 게시글 번역 기능에서 LLM의 오역 가능성을 줄이기 위해, Markdown 내 HTML 주석을 활용한 특별한 지침 제공 방법을 소개합니다. 게시글 상단에 `<!--`와 `-->` 사이에 LLM 번역가를 위한 지침을 작성하여 제목 지정, 특정 용어 사용 등의 구체적인 요청을 할 수 있습니다. 이는 LLM에게 맥락을 제공하여 번역 품질을 향상시키는 데 도움을 줍니다. 저자가 직접 번역 맥락을 설정함으로써 LLM 번역의 정확성을 높일 수 있다는 점에서 유용한 팁입니다.
Read more →새로 오신 분들도 많이 계시니, #자기소개 한 번 해 볼까요? 저부터 해보겠습니다.
- Hackers' Pub을 만들고 운영하고 있습니다. (Hackers' Pub은 저희 집 홈 서버에서 돌아가고 있습니다… 😂)
- 연합우주(fediverse)와 ActivityPub에 관심이 많고, 또 관련된 소프트웨어(
@fedifyFedify: an ActivityPub server framework,
@holloHollo
,
@botkitBotKit by Fedify
, Hackers' Pub…)를 만듭니다.
- 좋아하는 언어는 Haskell인데 자주 쓰는 언어는 TypeScript입니다. 예전에는 Python을 좋아하고 자주 썼습니다.
- 함수형 프로그래밍을 좋아합니다만, 좋아하는 만큼 잘 다루는지는 잘 모르겠습니다.
- 옛날에는 덕 타이핑 언어를 좋아했는데, 나이가 들고 협업을 많이 하게 되면서 정적 타이핑 언어를 선호하게 되었습니다. 그래도 여전히 덕 타이핑 언어가 제공하는 특별한 생산성이 있다고 생각합니다.
- 자유 소프트웨어와 오픈 소스를 좋아합니다. GPL을 좋아하지만, 트랜스젠더 배제적인 행보를 보인 적 있는 자유 소프트웨어 재단이나 여러 성추행 전적이 있는 Richard Stallman은 좋아하지 않습니다.
- 소프트웨어 문서화에도 관심이 많습니다. 문서화가 소프트웨어 개발의 중요한 부분이라고 생각합니다. 문서화 도구들에도 관심이 많습니다.
- 원래는 백엔드 개발자였는데 바로 전 직장이 블록체인 회사여서 백엔드 개발에서 손을 놓은 지 좀 되니까 이제는 잘 모르게 됐습니다. 재활이 필요합니다.
- 현재는 일 안 하고 쉬고 있습니다.
- 30대 후반, 기혼, 자녀는 없습니다. 서울에서 살고 있습니다.
아무쪼록 잘 부탁드립니다.
여기선 프로그래밍 언어론 이야기나 해야겠다
XiNiHa shared the below article:
“조용한 연합우주” 문제를 해결하는 두 가지 접근법: 대화 백필링 메커니즘
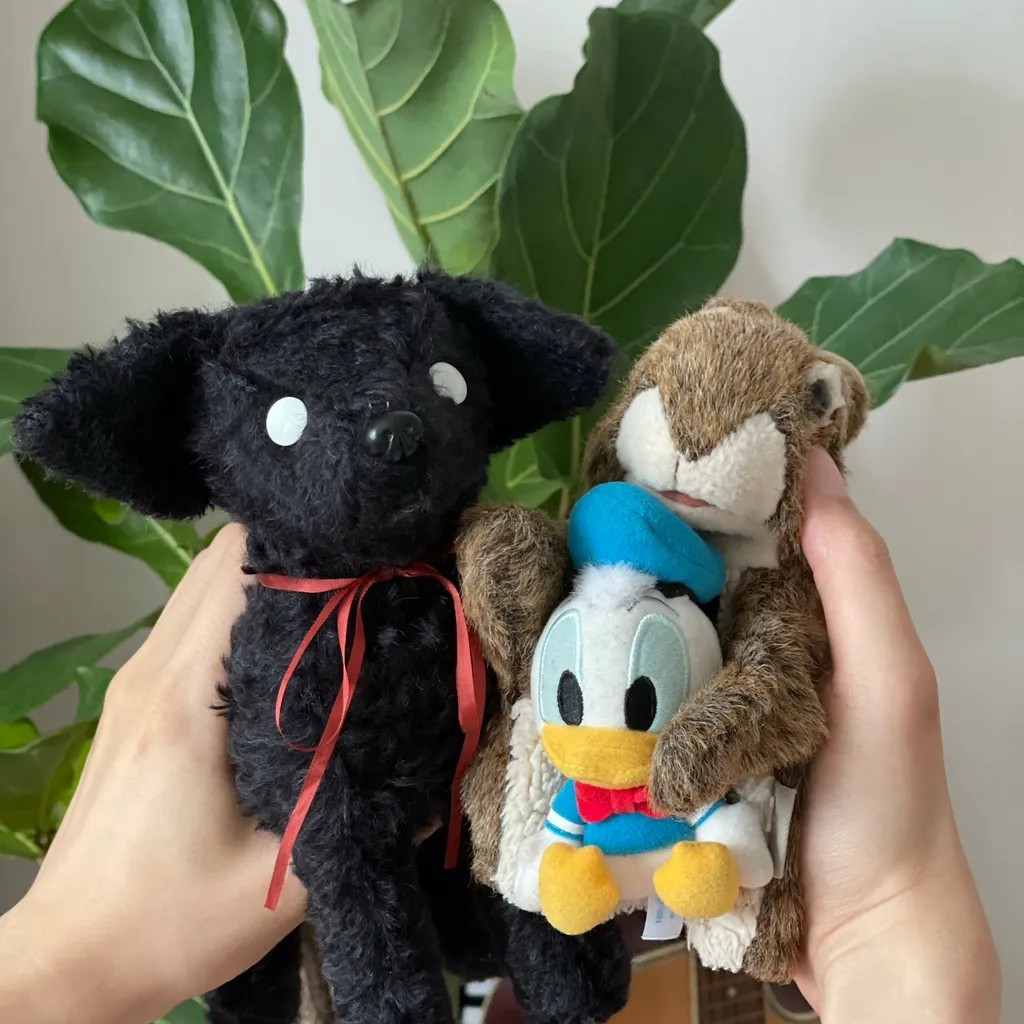
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
이 글은 연합우주(fediverse)에서 발생하는 "조용한 연합우주" 문제, 즉 대화의 일부만 보이는 현상의 원인과 해결책을 탐구합니다. ActivityPub 프로토콜의 분산 특성으로 인해 대화가 여러 서버에 분산되어 저장되면서 발생하는 이 문제를 해결하기 위해, 답글 트리 크롤링과 컨텍스트 소유자 기반 방식이라는 두 가지 주요 접근법을 제시합니다. 답글 트리 크롤링은 모든 답글을 순차적으로 가져오는 방식이지만 네트워크 취약성과 작업량 증가의 단점이 있고, 컨텍스트 소유자 방식은 대화의 원 작성자가 대화 내용을 관리하는 중앙화된 접근법이지만 컨텍스트 소유자에 대한 의존성이 높다는 단점이 있습니다. 또한, 모더레이션 패러다임의 충돌과 상위 전파 누락 문제와 같은 논쟁점을 지적하며, 주기적 크롤링, 사용자 트리거, 멘션 기반 백필과 같은 추가적인 백필 메커니즘을 소개합니다. 마지막으로, FEP 수렴 논의와 구현체 간 협력 현황을 통해 향후 개발 방향으로 하이브리드 접근법의 표준화를 제시하며, 다중 전략 구현, 리소스 관리, 모니터링 및 로깅의 모범 사례 가이드라인을 제시합니다. 이 글은 연합우주가 더욱 풍부하고 연결된 소셜 네트워크로 발전하기 위한 노력과 사용자 경험 개선의 중요성을 강조합니다.
Read more →평소에 함수형 언어 매니아들이 주장하는만큼 이펙트를 엄격하게 구분하는게 중요하다곤 생각안했는데, local first 앱을 만들다가 네트워크 요청을 포함한 IO와 그렇지 않은 IO를 구분해야하는 이유를 찾았다. 앱의 초기화 로직에 네트워크 요청이 숨어있으면 API 서버 장애시 앱이 아예 안켜지는 문제가 있다. 방금 이거랑 관련된 버그 찾느라 시간을 많이 썼다.
[페미위키 6월 운영팀 모집]
운영팀은 페미위키를 어떻게 키워낼까 고민하는 개인들의 모임이며 여성혐오자가 아니라면 추가 조건 없이 함께할 수 있습니다.
운영팀 연락에 사용할 구글 지메일로 admin@femiwiki.com으로 지원 동기를 적어 보내주세요. (제목 "페미위키 운영팀 지원")
안녕하세요, 페미위키 개발팀입니다. 개발팀 활성화를 위해 이리저리 둘러보다 해커스펍에 대해 알게 되었습니다. 여건이 되면 페미위키 개발에 대해서 얘기할 수 있는 기회를 만들어보려 합니다!
더불어 페미위키 개발팀에서 오픈소스 컨트리뷰터 & 개발팀을 모집합니다! 페미니스트시라면 정체성 불문, 거주국 불문하고 모시고 있습니다. 함께 페미니즘 정보집합체 만들어가요!
오늘 @xiniha 님 소환해서 배운 것:
- SolidStart 기본적인 사용법
- SolidStart 위에서 GraphQL 질의해서 결과 갖다 쓰는 법
- GraphQL + Relay에서 커넥션에 추가 필드 끼워넣는 법
- SolidStart에서 shadcn/ui…가 아니라 solid-ui 쓰는 법
그리고 배운 건 아니고 그냥 @xiniha 님이 다 해주신 것:
- Lingui를 이용한 국제화 세팅
- Deno를 쓰기 때문에 생기는 온갖 트러블들 해결
이제 이 새로운 스택으로 Hackers' Pub을 재구현하기만 하면 된다…! 다행히 도메인 모델은 분리되어 있어서, UI 위주로 재작성하면 될 것 같다.
We're migrating Hackers' Pub to a pretty unconventional tech stack, and I'm honestly excited about it!
Thanks to my friend @xiniha, we're diving into #Solid, #SolidStart, #Pothos, #GraphQL, and #Relay. In a world dominated by Next.js and React, this feels refreshingly different. And yes, we're sticking with #Deno instead of Node.js too.
Some might call it contrarian, but I like to think of it as exploring what's possible beyond the mainstream. Sometimes the road less traveled leads to interesting places.
Zed 설정 파일 형식은 VS Code보다 구조화도 덜 되어있고 쓰기에도 불편한 것 같다. 주먹구구식으로 만든 느낌…
@hongminhee洪 民憙 (Hong Minhee) 그래도 설정 파일 스키마가 바뀌었을 때 자동 업그레이드 지원하는 기능이 있어서, 당장 대충 만들어둬도 크게 문제는 없을 것 같긴 하더라구요 😂
Hackers' Pub에 공유할 수 있는 초대 링크 기능을 추가했습니다. 설정 → 초대 페이지에서 초대 링크 생성이 가능하며, 초대 링크는 정원이나 유효 기간, 메시지를 정할 수 있게 되어 있습니다. 아무래도 DM으로 직접 초대 요청을 달라고 하면 쑥스러움이 많은 분들은 요청을 안 하시는 경우가 많은 것 같아서 만들게 되었습니다. 참고로, 초대 링크를 통해 가입한 사용자는 초대 링크를 생성한 사람과 자동으로 맞팔하게 됩니다.
We're excited to announce Hollo 0.6.0, a significant release that brings enhanced security, better user experience, and important infrastructure improvements to your single-user microblogging setup.
Enhanced OAuth Security with Modern Standards
This release prioritizes security with comprehensive OAuth 2.0 improvements that align with current best practices. We've implemented several critical RFC standards that significantly strengthen the authorization process:
OAuth 2.0 Authorization Code Flow with Access Grants — We've overhauled the OAuth implementation to properly separate authorization codes from access token issuance, providing better security isolation throughout the authentication process.
RFC 7636 PKCE (Proof Key for Code Exchange) Support — Hollo now supports PKCE with the S256 code challenge method, which prevents authorization code interception attacks. This is particularly important for public clients and follows the latest OAuth 2.0 security recommendations outlined in RFC 9700 (OAuth 2.0 Security Current Best Practices).
RFC 8414 OAuth Authorization Server Metadata — We've added support for OAuth Authorization Server metadata endpoints, allowing clients to automatically discover Hollo's OAuth capabilities and configuration. This makes integration smoother and helps clients adapt to your server's specific OAuth setup.
Enhanced Profile Scope Support — The new /oauth/userinfo
endpoint and expanded profile scope support provide applications with standardized ways to access user profile information, improving compatibility with a wider range of OAuth-compliant applications.
These OAuth improvements not only make Hollo more secure but also position it at the forefront of federated social media security standards. We encourage other fediverse projects to adopt these same standards to ensure the entire ecosystem benefits from these security enhancements.
Special thanks to Emelia Smith (@thisismissemEmelia 👸🏻) for spearheading these critical OAuth security improvements and ensuring Hollo stays ahead of the curve on authentication best practices.
Revamped Media Storage Configuration
We've significantly improved how Hollo handles media storage configuration, making it more flexible and future-ready:
New Environment Variables — The storage system now uses STORAGE_URL_BASE
(replacing the deprecated ASSET_URL_BASE
) and FS_STORAGE_PATH
for local filesystem storage (replacing FS_ASSET_PATH
). These changes provide clearer naming and better organization.
Improved Security Requirements — The SECRET_KEY
environment variable now requires a minimum of 44 characters, ensuring sufficient entropy for cryptographic operations. You'll need to update your configuration if your current secret key is shorter.
Network Binding Control — The new BIND
environment variable lets you specify exactly which network interface Hollo should listen on, giving you more control over your server's network configuration.
Thanks to Emelia Smith (@thisismissemEmelia 👸🏻) for leading these infrastructure improvements.
Better User Experience
Customizable Profile Themes — You can now personalize your profile page with different theme colors. Choose from the full range of Pico CSS color options to make your profile uniquely yours.
Enhanced Administration Dashboard — The dashboard now displays the current Hollo version at the bottom, making it easier to track which version you're running. You can also sign out directly from the dashboard for better session management.
Improved Post Presentation — Shared posts on profile pages now have better visual separation from original content, and the sharing timestamp is clearly displayed. This makes it much easier to distinguish between your original thoughts and content you've shared from others.
Better Image Accessibility — Alt text for images is now displayed within expandable details sections, improving accessibility while keeping the interface clean.
Syntax Highlighting — Code blocks in Markdown posts now feature beautiful syntax highlighting powered by Shiki, supporting a comprehensive range of programming languages. This makes technical discussions much more readable.
Enhanced Character Limit — The maximum post length has been increased from 4,096 to 10,000 characters, giving you more space to express your thoughts in detail.
Thanks to RangHo Lee (@rangho_220우주스타 아이도루 랭호 🌠) for the version display feature and Okuto Oyama (
@yamanoku) for the image accessibility improvements.
Privacy and Content Improvements
EXIF Metadata Removal — Hollo now automatically strips EXIF metadata from uploaded images before storing them, protecting your privacy by removing potentially sensitive location and device information.
Public API Endpoints — Following Mastodon's approach, certain API endpoints are now publicly accessible without authentication, making Hollo more compatible with various client applications and improving the overall federation experience.
Thanks to NTSK (@ntekNTSK) for the privacy-focused EXIF metadata stripping implementation.
Technical Foundation
Node.js 24+ Requirement — This release requires Node.js 24.0.0 or later. We've also upgraded to Fedify 1.5.3 and @fedify/postgres 0.3.0 for improved performance and compatibility.
Test Coverage & Quality Assurance — The codebase now includes comprehensive testing infrastructure and test coverage. We're committed to expanding this coverage and integrating testing more deeply into our development and release workflows. This also provides an excellent opportunity for first-time contributors to get involved by writing tests.
Cross-Origin Request Support — OAuth and well-known endpoints now properly support cross-origin requests, aligning with Mastodon's behavior and improving client compatibility.
Cleaner Token Endpoint — The scope parameter is now properly optional for the OAuth token endpoint, clarifying that it only affects client credentials flows (not authorization code flows, where it was already ignored).
Looking Forward
This release represents a major step forward in making Hollo not just a great single-user microblogging platform, but also a leader in federated social media security standards. The OAuth improvements we've implemented should serve as a model for other fediverse projects.
We're particularly excited about the OAuth security enhancements, which demonstrate our commitment to staying ahead of security best practices. As the federated web continues to evolve, we believe these standards will become increasingly important for maintaining user trust and ensuring secure interactions across the fediverse.
Upgrading
Upgrading to Hollo 0.6.0 is straightforward, but there are a few important considerations:
Railway Deployment
- Go to your Railway dashboard
- Select your Hollo project and service
- In the deployments tab, click the three-dot menu and select Redeploy
Docker Deployment
- Pull the latest image:
docker pull ghcr.io/fedify-dev/hollo:latest
- Stop your current container
- Start with the new image using your existing configuration
Manual Installation
- Pull the latest code:
git pull
- Install dependencies:
pnpm install
- Restart the service:
pnpm run prod
Important Upgrade Notes
Environment Variables: Update your configuration if you're using deprecated variables:
- Replace
ASSET_URL_BASE
withSTORAGE_URL_BASE
- Replace
FS_ASSET_PATH
withFS_STORAGE_PATH
- Ensure your
SECRET_KEY
is at least 44 characters long
Session Reset: Due to the OAuth security improvements, existing user sessions may be invalidated during the upgrade. You'll likely need to log in again through your client apps (like Phanpy, Moshidon, etc.) after upgrading. This is a one-time inconvenience that ensures you benefit from the enhanced security features.
Thank you to everyone who contributed to this release, and to the community for your continued support. Hollo 0.6.0 brings significant improvements to security, usability, and the overall experience of running your own corner of the fediverse.
Exciting news for the #Hollo project! We're thrilled to announce that Emelia Smith (@thisismissemEmelia 👸🏻) has joined as a co-maintainer alongside Hong Minhee (
@hongminhee洪 民憙 (Hong Minhee)).
Emelia brings extensive experience in the #fediverse ecosystem, having been a long-time contributor to Mastodon and a leading expert in trust & safety tooling for decentralized social networks. She's dedicated years to improving moderation systems and security across #ActivityPub platforms.
Her recent contributions to Hollo have been substantial—implementing the reporting/flagging system and making significant improvements to OAuth and security features. These valuable contributions naturally led to her joining as a co-maintainer.
This collaboration marks an important milestone for Hollo as we continue building better single-user microblogging software for the fediverse. Welcome aboard, Emelia! 🚀
Hackers' Pub을 사용하면서 연합우주(fediverse) 뿐만 아니라 Bluesky 사람들과도 교류하고 싶으신 분들은 Bridgy Fed라는 서비스를 사용해 보시면 좋을 것 같습니다. Hackers' Pub 계정 생성 후 2주가 지난 분들만 사용 가능하긴 한데요.[1]
@bsky.brid.gyBridgy Fed for Bluesky 계정을 팔로하시면 Bluesky 쪽에 일종의 미러링 계정이 생성되게 됩니다. 성공적으로 Bluesky 미러가 생기면
@bsky.brid.gyBridgy Fed for Bluesky 계정이 맞팔을 해 올 겁니다.
예를 들어 제 @hongminhee洪 民憙 (Hong Minhee) 계정으로
@bsky.brid.gyBridgy Fed for Bluesky 계정을 팔로하면, Bluesky 쪽에 @hongminhee.hackers.pub.ap.brid.gy라는 계정이 생기는 식입니다. 그러면 Bluesky 쪽 사람들이 해당 계정을 멘션하거나, 댓글을 달거나, 인용을 하면 Hackers' Pub에서 그게 보이게 됩니다. 서로 팔로도 할 수 있고요.