해커스펍이 죽은 건지 내 탐라가 죽은 건지 해커스펍이 정전인 건지 내 탐라가 정전인 건지
XiNiHa
@xiniha@hackers.pub · 79 following · 91 followers
GitHub
- @XiNiHa
Bluesky
- @xiniha.dev
Twitter
- @xiniha_1e88df
음 그냥 아무도 글을 안 쓴 게 맞는 것 같구만
해커스펍이 죽은 건지 내 탐라가 죽은 건지 해커스펍이 정전인 건지 내 탐라가 정전인 건지
XiNiHa shared the below article:
Ditch the DIY Drama: Why Use Fedify Instead of Building ActivityPub from Scratch?
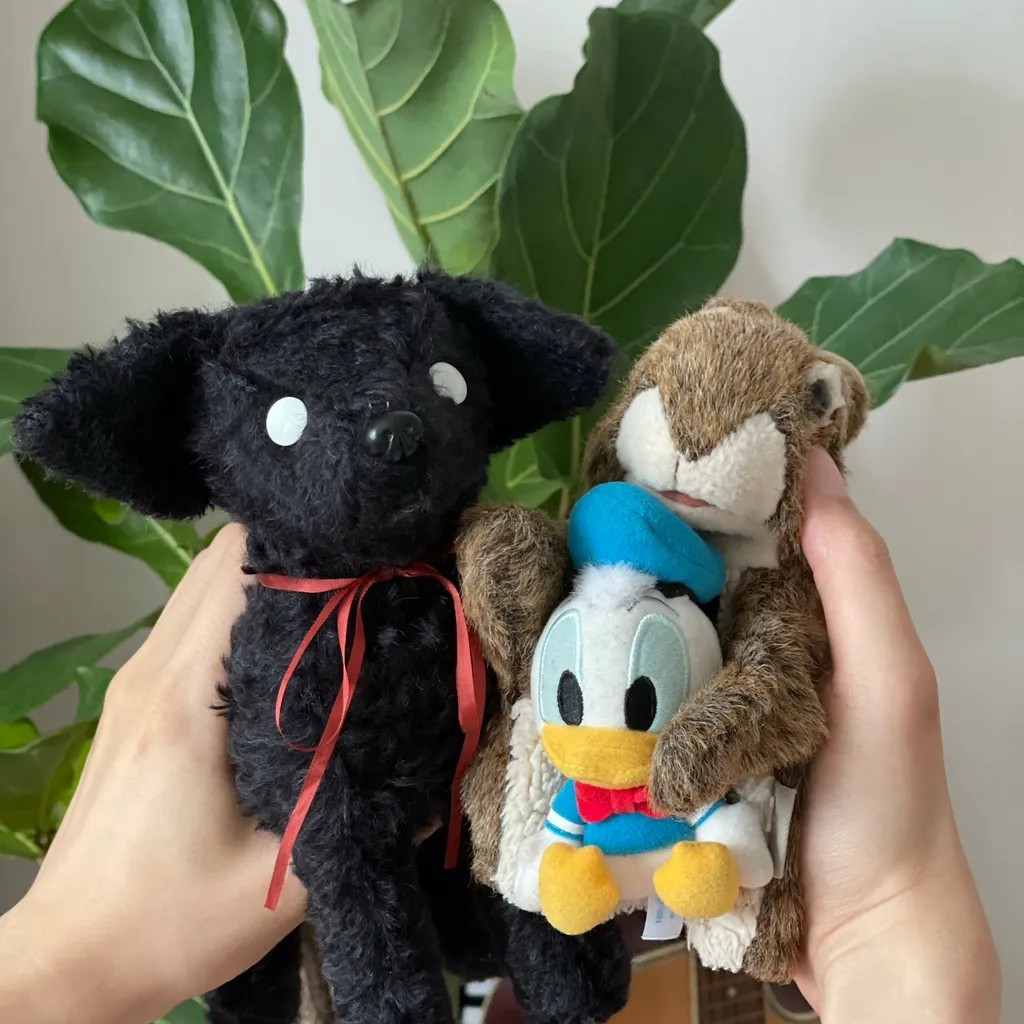
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
So, you're captivated by the fediverse—the decentralized social web powered by protocols like ActivityPub. Maybe you're dreaming of building the next great federated app, a unique space connected to Mastodon, Lemmy, Pixelfed, and more. The temptation to dive deep and implement ActivityPub yourself, from the ground up, is strong. Total control, right? Understanding every byte? Sounds cool!
But hold on a sec. Before you embark on that epic quest, let's talk reality. Implementing ActivityPub correctly isn't just one task; it's like juggling several complex standards while riding a unicycle… blindfolded. It’s hard.
That's where Fedify comes in. It's a TypeScript framework designed to handle the gnarliest parts of ActivityPub development, letting you focus on what makes your app special, not reinventing the federation wheel.
This post will break down the common headaches of DIY ActivityPub implementation and show how Fedify acts as the super-powered pain reliever, starting with the very foundation of how data is represented.
Challenge #1: Data Modeling—Speaking ActivityStreams & JSON-LD Fluently
At its core, ActivityPub relies on the ActivityStreams 2.0 vocabulary to describe actions and objects, and it uses JSON-LD as the syntax to encode this vocabulary. While powerful, this combination introduces significant complexity right from the start.
First, understanding and correctly using the vast ActivityStreams vocabulary itself is a hurdle. You need to model everything—posts (Note
, Article
), profiles (Person
, Organization
), actions (Create
, Follow
, Like
, Announce
)—using the precise terms and properties defined in the specification. Manual JSON construction is tedious and prone to errors.
Second, JSON-LD, the encoding layer, has specific rules that make direct JSON manipulation surprisingly tricky:
- Missing vs. Empty Array: In JSON-LD, a property being absent is often semantically identical to it being present with an empty array. Your application logic needs to treat these cases equally when checking for values. For example, these two
Note
objects mean the same thing regarding thename
property:// No name property { "@context": "https://www.w3.org/ns/activitystreams", "type": "Note", "content": "…" }
// Equivalent to: { "@context": "https://www.w3.org/ns/activitystreams", "type": "Note", "name": [], "content": "…" }
- Single Value vs. Array: Similarly, a property holding a single value directly is often equivalent to it holding a single-element array containing that value. Your code must anticipate both representations for the same meaning, like for the
content
property here:// Single value { "@context": "https://www.w3.org/ns/activitystreams", "type": "Note", "content": "Hello" }
// Equivalent to: { "@context": "https://www.w3.org/ns/activitystreams", "type": "Note", "content": ["Hello"] }
- Object Reference vs. Embedded Object: Properties can contain either the full JSON-LD object embedded directly or just a URI string referencing that object. Your application needs to be prepared to fetch the object's data if only a URI is given (a process called dereferencing). These two
Announce
activities are semantically equivalent (assuming the URIs resolve correctly):{ "@context": "https://www.w3.org/ns/activitystreams", "type": "Announce", // Embedded objects: "actor": { "type": "Person", "id": "http://sally.example.org/", "name": "Sally" }, "object": { "type": "Arrive", "id": "https://sally.example.com/arrive", /* ... */ } }
// Equivalent to: { "@context": "https://www.w3.org/ns/activitystreams", "type": "Announce", // URI references: "actor": "http://sally.example.org/", "object": "https://sally.example.com/arrive" }
Attempting to manually handle all these vocabulary rules and JSON-LD variations consistently across your application inevitably leads to verbose, complex, and fragile code, ripe for subtle bugs that break federation.
Fedify tackles this entire data modeling challenge with its comprehensive, type-safe Activity Vocabulary API. It provides TypeScript classes for ActivityStreams types and common extensions, giving you autocompletion and compile-time safety. Crucially, these classes internally manage all the tricky JSON-LD nuances. Fedify's property accessors present a consistent interface—non-functional properties (like tags
) always return arrays, functional properties (like content
) always return single values or null. It handles object references versus embedded objects seamlessly through dereferencing accessors (like activity.getActor()
) which automatically fetch remote objects via URI when needed—a feature known as property hydration. With Fedify, you work with a clean, predictable TypeScript API, letting the framework handle the messy details of AS vocabulary and JSON-LD encoding.
Challenge #2: Discovery & Identity—Finding Your Actors
Once you can model data, you need to make your actors discoverable. This primarily involves the WebFinger protocol (RFC 7033). You'd need to build a server endpoint at /.well-known/webfinger
capable of parsing resource queries (like acct:
URIs), validating the requested domain against your server, and responding with a precisely formatted JSON Resource Descriptor (JRD). This JRD must include specific links, like a self
link pointing to the actor's ActivityPub ID using the correct media type. Getting any part of this wrong can make your actors invisible.
Fedify simplifies this significantly. It automatically handles WebFinger requests based on the actor information you provide through its setActorDispatcher()
method. Fedify generates the correct JRD response. If you need more advanced control, like mapping user-facing handles to internal identifiers, you can easily register mapHandle()
or mapAlias()
callbacks. You focus on defining your actors; Fedify handles making them discoverable.
// Example: Define how to find actors
federation.setActorDispatcher(
"/users/{username}",
async (ctx, username) => { /* ... */ }
);
// Now GET /.well-known/webfinger?resource=acct:username@your.domain just works!
Challenge #3: Core ActivityPub Mechanics—Handling Requests and Collections
Serving actor profiles requires careful content negotiation. A request for an actor's ID needs JSON-LD for machine clients (Accept: application/activity+json
) but HTML for browsers (Accept: text/html
). Handling incoming activities at the inbox endpoint involves validating POST
requests, verifying cryptographic signatures, parsing the payload, preventing duplicates (idempotency), and routing based on activity type. Implementing collections (outbox
, followers
, etc.) with correct pagination adds another layer.
Fedify streamlines all of this. Its core request handler (via Federation.fetch()
or framework adapters like @fedify/express) manages content negotiation. You define actors with setActorDispatcher()
and web pages with your framework (Hono, Express, SvelteKit, etc.)—Fedify routes appropriately. For the inbox, setInboxListeners()
lets you define handlers per activity type (e.g., .on(Follow, ...)
), while Fedify automatically handles validation, signature verification, parsing, and idempotency checks using its KV Store. Collection implementation is simplified via dispatchers (e.g., setFollowersDispatcher()
); you provide logic to fetch a page of data, and Fedify constructs the correct Collection
or CollectionPage
with pagination.
// Define inbox handlers
federation.setInboxListeners("/inbox", "/users/{handle}/inbox")
.on(Follow, async (ctx, follow) => { /* Handle follow */ })
.on(Undo, async (ctx, undo) => { /* Handle undo */ });
// Define followers collection logic
federation.setFollowersDispatcher(
"/users/{handle}/followers",
async (ctx, handle, cursor) => { /* ... */ }
);
Challenge #4: Reliable Delivery & Asynchronous Processing—Sending Activities Robustly
Sending an activity requires more than a simple POST
. Networks fail, servers go down. You need robust failure handling and retry logic (ideally with backoff). Processing incoming activities synchronously can block your server. Efficiently broadcasting to many followers (fan-out) requires background processing and using shared inboxes where possible.
Fedify addresses reliability and scalability using its MessageQueue
abstraction. When configured (highly recommended), Context.sendActivity()
enqueues delivery tasks. Background workers handle sending with automatic retries based on configurable policies (like outboxRetryPolicy
). Fedify supports various queue backends (Deno KV, Redis, PostgreSQL, AMQP). For high-traffic fan-out, Fedify uses an optimized two-stage mechanism to distribute the load efficiently.
// Configure Fedify with a persistent queue (e.g., Deno KV)
const federation = createFederation({
queue: new DenoKvMessageQueue(/* ... */),
// ...
});
// Sending is now reliable and non-blocking
await ctx.sendActivity({ handle: "myUser" }, recipient, someActivity);
Challenge #5: Security—Avoiding Common Pitfalls
Securing an ActivityPub server is critical. You need to implement HTTP Signatures (draft-cavage-http-signatures-12) for server-to-server authentication—a complex process. You might also need Linked Data Signatures (LDS) or Object Integrity Proofs (OIP) based on FEP-8b32 for data integrity and compatibility. Managing cryptographic keys securely is essential. Lastly, fetching remote resources risks Server-Side Request Forgery (SSRF) if not validated properly.
Fedify is designed with security in mind. It automatically handles the creation and verification of HTTP Signatures, LDS, and OIP, provided you supply keys via setKeyPairsDispatcher()
. It includes key management utilities. Crucially, Fedify's default document loader includes built-in SSRF protection, blocking requests to private IPs unless explicitly allowed.
Challenge #6: Interoperability & Maintenance—Playing Nicely with Others
The fediverse is diverse. Different servers have quirks. Ensuring compatibility requires testing and adaptation. Standards evolve with new Federation Enhancement Proposals (FEPs). You also need protocols like NodeInfo to advertise server capabilities.
Fedify aims for broad interoperability and is actively maintained. It includes features like ActivityTransformer
s to smooth over implementation differences. NodeInfo support is built-in via setNodeInfoDispatcher()
.
Challenge #7: Developer Experience—Actually Building Your App
Beyond the protocol, building any server involves setup, testing, and debugging. With federation, debugging becomes harder—was the message malformed? Was the signature wrong? Is the remote server down? Is it a compatibility quirk? Good tooling is essential.
Fedify enhances the developer experience significantly. Being built with TypeScript, it offers excellent type safety and editor auto-completion. The fedify
CLI is a powerful companion designed to streamline common development tasks.
You can quickly scaffold a new project tailored to your chosen runtime and web framework using fedify init
.
For debugging interactions and verifying data, fedify lookup
is invaluable. It lets you inspect how any remote actor or object appears from the outside by performing WebFinger discovery and fetching the object's data. Fedify then displays the parsed object structure and properties directly in your terminal. For example, running:
$ fedify lookup @fedify-example@fedify-blog.deno.dev
Will first show progress messages and then output the structured representation of the actor, similar to this:
// Output of fedify lookup command (shows parsed object structure)
Person {
id: URL "https://fedify-blog.deno.dev/users/fedify-example",
name: "Fedify Example Blog",
published: 2024-03-03T13:18:11.857Z, // Simplified timestamp
summary: "This blog is powered by Fedify, a fediverse server framework.",
url: URL "https://fedify-blog.deno.dev/",
preferredUsername: "fedify-example",
publicKey: CryptographicKey {
id: URL "https://fedify-blog.deno.dev/users/fedify-example#main-key",
owner: URL "https://fedify-blog.deno.dev/users/fedify-example",
publicKey: CryptoKey { /* ... CryptoKey details ... */ }
},
// ... other properties like inbox, outbox, followers, endpoints ...
}
This allows you to easily check how data is structured or troubleshoot why an interaction might be failing by seeing the actual properties Fedify parsed.
Testing outgoing activities from your application during development is made much easier with fedify inbox
. Running the command starts a temporary local server that acts as a publicly accessible inbox, displaying key information about the temporary actor it creates for receiving messages:
$ fedify inbox
✔ The ephemeral ActivityPub server is up and running: https://<unique_id>.lhr.life/
✔ Sent follow request to @<some_test_account>@activitypub.academy.
╭───────────────┬─────────────────────────────────────────╮
│ Actor handle: │ i@<unique_id>.lhr.life │
├───────────────┼─────────────────────────────────────────┤
│ Actor URI: │ https://<unique_id>.lhr.life/i │
├───────────────┼─────────────────────────────────────────┤
│ Actor inbox: │ https://<unique_id>.lhr.life/i/inbox │
├───────────────┼─────────────────────────────────────────┤
│ Shared inbox: │ https://<unique_id>.lhr.life/inbox │
╰───────────────┴─────────────────────────────────────────╯
Web interface available at: http://localhost:8000/
You then configure your developing application to send an activity to the Actor inbox
or Shared inbox
URI provided. When an activity arrives, fedify inbox
only prints a summary table to your console indicating that a request was received:
╭────────────────┬─────────────────────────────────────╮
│ Request #: │ 2 │
├────────────────┼─────────────────────────────────────┤
│ Activity type: │ Follow │
├────────────────┼─────────────────────────────────────┤
│ HTTP request: │ POST /i/inbox │
├────────────────┼─────────────────────────────────────┤
│ HTTP response: │ 202 │
├────────────────┼─────────────────────────────────────┤
│ Details │ https://<unique_id>.lhr.life/r/2 │
╰────────────────┴─────────────────────────────────────╯
Crucially, the detailed information about the received request—including the full headers (like Signature
), the request body (the Activity JSON), and the signature verification status—is only available in the web interface provided by fedify inbox
. This web UI allows you to thoroughly inspect incoming activities during development.

When you need to test interactions with the live fediverse from your local machine beyond just sending, fedify tunnel
can securely expose your entire local development server temporarily. This suite of tools significantly eases the process of building and debugging federated applications.
Conclusion: Build Features, Not Plumbing
Implementing the ActivityPub suite of protocols from scratch is an incredibly complex and time-consuming undertaking. It involves deep dives into multiple technical specifications, cryptographic signing, security hardening, and navigating the nuances of a diverse ecosystem. While educational, it dramatically slows down the process of building the actual, unique features of your federated application.
Fedify offers a well-architected, secure, and type-safe foundation, handling the intricacies of federation for you—data modeling, discovery, core mechanics, delivery, security, and interoperability. It lets you focus on your application's unique value and user experience. Stop wrestling with low-level protocol details and start building your vision for the fediverse faster and more reliably. Give Fedify a try!
Getting started is straightforward. First, install the Fedify CLI using your preferred method. Once installed, create a new project template by running fedify init your-project-name
.
Check out the Fedify tutorials and Fedify manual to learn more. Happy federating!
드디어 @xtjuxtapose 님이 기다리시던 차단 기능이 구현되었습니다. 차단할 사용자의 프로필 페이지에 가서 팔로·언팔로 버튼 오른쪽에 보이는 말줄임표 아이콘에 마우스 커서를 갖다 대면 (모바일에서는 터치하면) 상세 메뉴가 나오는데, 그 안에 팔로워 삭제 버튼과 차단 버튼이 생겼습니다.
ActivityPub 프로토콜 수준에서는 차단은 Block
액티비티를 차단한 액터에게 보내며, 차단을 해제할 경우 Undo(Block)
액티비티를 보냅니다. 그러나, 그 액티비티를 받은 인스턴스의 구현이 차단한 사용자의 콘텐츠를 볼 수 없도록 막지 않을 수도 있습니다…만, 실질적으로는 모든 구현이 막고 있습니다. 아, 당연하지만 차단은 자동적으로 상호 언팔로를 수행합니다. 차단을 해제하더라도 풀렸던 팔로 관계는 자동적으로 회복되지 않습니다.
개인적으로는 k8s쓰는 가장 큰 이유는 개발자 복지라고 생각한다. 적정기술만 쓰면 대부분의 사람들은 뭔가를 실 서비스에서 경험할 기회를 잃어버린다. 아니 이건 됐고…
온프레미스 클러스터 오퍼레이션 부담이나 EKS같은 서비스의 사용료 걱정만 없다면 쓰는게 무조건 낫다고 생각한다.
일단 k8s뿐만 아니라 컨테이너/머신 오케스트레이션의 세계에서 앱과 머신은 좀 더 잘 죽어도되는 존재가 된다. (물론 stateful한 호스트와 앱을 최대한 stateless하게 하거나, 상태를 분리하여 격리시켜야 하긴 한다)
그러면 docker-compose로 충분하지 않느냐 말할 사람도 있겠지만 처음에야 docker-compose 쓰는거나 k8s 쓰는거나 그게 그거지만(오히려 k8s가 성가실것이다) 마이그레이션의 때가 오면 난 그걸 감당할 자신이 없다.
물론 자신만의 가볍고 쏙 맘에드는 솔루션을 고집할 사람도 있을텐데… 난 남들이 다 쓰는거 쓰는게 편하다.
v1의 구현방식이 매력적이었는데 한계로 인해 결국 v2의 방식을 택하게된게 참 아쉬웠다…
RE: https://hl.pkgu.net/@pkgupdt/019627a1-2be1-763a-90e2-1e10ce1df445
패디버스 앱으로서 해커스펍이 누릴 수 있는 기능 중 하나가 Remote Follow인데, 이건 다른 페디버스 앱에서도 마찬가지로 이런 기능이라는게 있다는걸 알지 못하는 경우가 많다. 새로 진입하는 분들 타겟으로 카드뉴스 같은거라도 만들어야 하나...... 라는 생각이 문득 들었다.
좋은 기능이 있어도 설명이 필요하다는 것 자체가 성공한 UX는 아닌 것 같은데, 이게 구조 상 어쩔 수 없이 생기는 문제인건가 싶기도 하고
2025年 오픈소스 컨트리뷰션 아카데미 參與型 멘토團 募集 公告가 떴다. Fedify 프로젝트의 메인테이너로서 멘토團에 志願하고자 한다. 志願書가 .hwp 파일이기에 큰 맘 먹고 한컴오피스 한글 for Mac도 購入했다. (아무래도 앞으로 .hwp 파일 다룰 일이 많을 것 같다는 豫感이 들어서…)
Hackers' Pub에 차단 기능을 만들다 보니 일단 모델 쪽에서 팔로워 삭제 기능이 필요하다는 것을 깨달았다. 즉시 하던 작업 다 git stash
하고 팔로워 삭제 먼저 만드는 중… (하지만 UI로 노출하는 건 나중에 할 생각.)
UI로 노출하는 건 나중에 만드려고 했지만, 어차피 테스트도 해야 해서 UI로도 노출했습니다. 자신의 팔로워 목록 페이지에 가시면 각 팔로워마다 오른쪽 위에 X 모양 아이콘이 생겼을 겁니다. 그걸 누르면 팔로워를 삭제할 수 있습니다. (X로 치면 “블언블”한 효과.)
협업을 할 때 미묘하게 거슬리는 것들
- 텍스트 파일의 마지막 줄 완성 안 하기 Posix 표준 기준으로 각 줄은 새줄 문자(line feed, '\n')로 끝나야 하며, 마지막 줄도 예외는 아님
- 그리고 위의 일이 주기적으로 재발할 때
쿠버네티스는 컴포즈만으로는 할 수 없는 HA (네트워킹 포함) 까지 책임져주는 솔루션 중 de-facto 라서 쓴다고 생각해요
앵간한 상황 다 대응되고
복잡하고 어려운 이유는 "앵간한 상황 다 대응"되기 때문이 아닐까 싶음... 컨테이너만 띄울거면 이렇게 복잡해질 이유가 없었음 - 사실 그 부분만 보면 컴포즈랑 비슷하기도 하고
@xiniha 오 어떤게 그렇게 나오는지 궁금합니다!
어찌보면 말씀하신걸 그대로 다른 솔루션으로 만들 수 있다는걸 알아서 더 그렇지 않을까... 라는 생각도 드는군요 :)
@ujuc우죽 일단 쿠버가 가지는 강점은 결국 vendor-neutral한 플랫폼 위에서 다양한 벤더들이 만든 서드파티 소프트웨어를 엮어서 각자가 선호하는 애플리케이션 배포 환경을 구축할 수 있다는 점이라고 보는데요, 물론 앱 걍 띄우면 되지 굳이 커스텀된 배포 환경을 구축할 필요성이 있냐 하는 입장이라면 쿠버가 분명한 오버킬이 되겠지만 😅 개인적으로는 홈서버만 굴리는 상황에서도 도커컴포즈나 systemd를 얼기설기 엮어서 매번 뭔가를 만들기보단 처음에 한번 쿠버로 이것저것 환경 구축해두고 나중에 앱 올릴 때는 Helm 차트 파라미터만 약간 수정해서 올리는 식으로 사용하는 쪽을 선호하게 되더라구요. 해당 장점을 온전하게 대체할 수 있는 메인스트림 플랫폼이 현재로썬 없는 것으로 보이다 보니 자연스레 쿠버네티스를 밀게 되는데, 중장기적으로 WASM 컴포넌트 생태계가 성장하게 되면 wasmCloud 같은 것들이 더 우아한 대체제로 떠오를 가능성도 크다고 생각합니다!
GeekNews의 〈소프트웨어 엔지니어로 산다는 건 미친 짓이야〉 주제에 @youknowone 님께서 쓰신 좋은 댓글:
소프트웨어 개발이 어려운 일이라는 사람들은 본인이 그 일을 하는 이유가 뭘까요? 고되고 힘든 일이지만 보람있는 일이라서 하시나요? 이 업계에서 그런 분들은 그리 많지는 않았던 것 같습니다. 남들이 못하는 것 같으니까 어렵다고 주장하는거지, 실상은 그게 본인한테 가장 쉬운 일이니까 하시는 것 아닌가요? 남들이 좀 띄워준다고 자화자찬하면서 나만 특별한 양 여기면서 눈을 가리지 말고 주위를 봐야합니다. 이공계에서 어떤 분야가 방구석에서 인터넷 좀 보고 독학한다고 (잘 하면) 몇달만에 현업에 투입할 수 있는 전문가가 됩니까?
(…중략…)
물론 남들이 가지지 못한 훌륭한 손재주를 가진 사람은 존중받아 마땅하지만, 약간의 손재주를 연마했다고 해서 소싯적 배워둔 손재주로 평생 먹고 살면 좋을텐데 왜 그럴수 없을까, 나는 이런 훌륭한 손재주를 가졌는데 다른 사람들처럼 힘들게 일하지 않아야 하는 것 아닐까, 나는 남들은 쉽게 하지 못하는 대단한 재능을 가진 것이 아닐까 등등의 특별한 나에 심취하는건 교만에 가까운 일이 아닐까 합니다.
⚛️📝 New on Overreacted: React for Two Computers
React for Two Computers — over...
PostgreSQL에서는 GENERATED ALWAYS AS
절을 통해 생성된 칼럼을 지원하는데, 이게 간단한 사칙연산이나 문자열 연결 정도는 쉽게 가능하지만, 이 안에서 서브쿼리를 쓸 수 없기 때문에 조금만 복잡한 계산도 할 수가 없다. 나 같은 경우에는 {"foo": 1, "bar": 2, "baz": 3}
과 같은 jsonb
값이 있을 때 이로부터 6
과 같은 합산을 해야 하는 상황인데, sum()
같은 집계 함수를 쓸 수 없기 때문에 원하는 동작을 만들어 내지 못한다… 최후의 카드로 커스텀 함수 정의해서 쓰는 방법이 있긴 한데… 음…
사실 애초부터 Drizzle ORM이 클라이언트에서 계산되는 칼럼 같은 기능을 제공해 주면 일이 이렇게 복잡하지 않을 것 같은데. 무슨 ORM이 게터(getter)를 정의할 수 없는 건지… 아니면 있는데 나만 모르고 있는 건가!?
메타적인 얘기지만, 저는 오늘 “Kubernetes는 적정 기술인가?”라는 떡밥이 Hackers' Pub 내부적으로 발생했다는 사실이 아주 기쁩니다. 여태까지는 Hackers' Pub 자체에 대한 떡밥이거나, GeekNews나 X에서 넘어온 떡밥이었거든요.
오늘부터 쿠버세를 걷겠다
똑같은 얘기를 닉스, 닉스오에스, 닉스옵스로도 할 수 있어요. "아 이거 걍 선언형으로 파일 작성하고 nixops deploy --check
하나만 때리면 알아서 이것저것 다 뜨고 다 설정될 텐데 괜히 귀찮게 왜 쿠버네티스를"
근데 현실적으로는 그냥 도커 컴포즈가 제일 삽질의 총량이 적죠...
@xtjuxtapose Nix는 제가 잘 아는 분야가 아니니 도커 컴포즈 쪽만 생각하고 이야기해보면.... 컴포즈는 쿠버네티스처럼 "편하게 이것저것 띄울 수 있는 플랫폼"이라고 보기에는 부족하거나 불편한 점이 꽤 많다고 느꼈습니다. 물론 앱 한두 개만 띄우고 말 거면 컴포즈가 훨씬 간단한 건 맞지만 개인적으로는 쿠버네티스의 플랫폼스러움이 주는 장점이 꽤나 크게 느껴지더라구요
개인적으로 쿠버네티스를 편하게 쓰기 위한 공부를 어느 정도 마친 상태에서 보면 "아 이거 쿠버 하나만 떠 있으면 편하게 이것저것 띄우고 할 수 있는데 괜히 귀찮게 세팅해야 하네"라는 생각이 들기도 하지만 😅 쿠버네티스 잘 모르는 상태에서는 참 막막하겠다 싶긴 합니다....
RE: https://hackers.pub/@xt/01961970-a29f-78ff-baaf-1db2056a78e1
저, 저도 90% 이상의 경우 도커 컴포즈 정도가 적당한 추상화 수준이라고 생각해요...
실제로 쿠버네티스 쓰는 팀에서 일해 본 경험도 그렇고, 주변 이야기 들어 봐도 그렇고, 도입하면 도입한 것으로 인해 증가하는 엔지니어링 코스트가 분명히 있다는 점은 누구도 부인하지 않는 것 같은데요. 쿠버네티스를 제대로 쓰는 것 자체도 배워야 할 것도 많고, 엔지니어가 유능해야 하고, 망치도 들여야 하고... 웬만하면 전담할 팀이 필요하지 않나 싶어요. (전담할 '사람' 한 명으로 때우기에는, 그 사람 휴가 가면 일이 마비되니까.)
엔지니어만 100명이 넘는 곳이라면 확실히 도입의 이득이 더 크겠지만, 반대로 혼자 하는 프로젝트라면 도무지 수지타산이 안 나올 것이라고 생각합니다. 따라서 쟁점은 그 손익분기점이 어디냐일 텐데... "대부분의" 서비스는 대성공하기 전까지는 도입 안 해도 되지 않나, 조심스럽게 말씀드려 봅니다. 즉 쿠버네티스가 푸는 문제는 마세라티 문제인 것이죠...
특히 클라우드 남의 컴퓨터 를 쓰지 않고 베어메탈 쓰는 경우는 더더욱...
RE: https://hackers.pub/@hongminhee/019618b4-4aa4-7a20-8e02-cd9fed50caae
Hackers' Pub의 에모지 반응 기능은 Mastodon의 좋아요, Misskey 계열, Pleroma 계열, kmyblue 및 Fedibird의 에모지 반응 기능과 호환됩니다. 기술적으로는 기본 에모지인 ❤️는 Like
액티비티로 표현되며 그 외 나머지 에모지는 EmojiReact
액티비티로 표현됩니다. Mastodon, kmyblue, Fedibird의 좋아요는 ❤️ 에모지 반응으로 변환됩니다 (Misskey의 동작과 유사). 또한, Misskey 계열과 달리 한 사람이 한 콘텐츠에 여러 에모지 반응을 남길 수도 있습니다 (Pleroma 계열의 동작과 유사). Hackers' Pub 사용자가 남길 수 있는 에모지 반응은 ❤️, 🎉, 😂, 😲, 🤔, 😢, 👀 이렇게 7종이며, 그 외의 에모지 및 커스텀 에모지는 보낼 수는 없고 받는 것만 됩니다.
에모지 반응 기능이 배포되었습니다. 당분간 버그가 좀 있을 수도 있지만 양해 바랍니다. 전 이제 차단을 구현하러 가겠습니다…
패치 릴리스할 때 되도록이면 많은 버그 수정을 하나의 릴리스에 담고 싶은 충동.
@hongminhee洪 民憙 (Hong Minhee) (소근소근) 보는 사람들은 대개 더 잘게 쪼갤수록 좋아합니다. 체인지로그 한 덩어리가 너무 길면 읽을 엄두가 안 나서요. 더 자주 릴리스하면 더 활발하고 더 건강한 프로젝트처럼 보이게 되는 것은 덤...
패치 릴리스할 때 되도록이면 많은 버그 수정을 하나의 릴리스에 담고 싶은 충동.
@xiniha 아악 이런 거 하지 마세욧
@hongminhee洪 民憙 (Hong Minhee) ㅋㅋㅋㅋㅋㅋㅋㅋㅋ
음 더 길어야 하나
그러네
문득 alt text가 매우 길어지면 UI 상으로 어떻게 보일지 궁금해짐
음 더 길어야 하나
문득 alt text가 매우 길어지면 UI 상으로 어떻게 보일지 궁금해짐
Vitest에 이상한 거 제안하는 중
코드:
test("streaming ssr", async () => {
const Comp = (props) => {
const data = createAsync(() => props.promise);
return (
<Suspense fallback="Loading...">
<span>Data: {data()}</span>
</Suspense>
);
};
const stream = await run$("server", async (ctx) => {
const { promise, resolve } = Promise.withResolvers();
ctx.set("resolve", resolve);
return renderToStream(() => <Comp promise={promise} />);
});
await run$("client", async () => {
hydrate(stream, document.body);
});
await expect$("client").element(page.getByText("Loading...")).toBeInTheDocument();
await run$("server", async (ctx) => {
const resolve = ctx.get("resolve");
resolve(42);
});
await expect$("client").element(page.getByText("Data: 42")).toBeInTheDocument();
await expect$("client").element(page.getByText("Loading...")).not.toBeInTheDocument();
})
Vitest에 이상한 거 제안하는 중
이게 맞나…?
@hongminhee洪 民憙 (Hong Minhee) 컼 너무 미어터지는데 깃허브처럼 별도 row로 두고 0은 숨기도록 하면 어떨까요
와 웹이라자주안들어왔었는데 이거은근잼있네 근데 나처럼 뻘소리 올리는사람이별로없어보임
@darkenpengpenguin 저도 뭔가 여기는 개발 얘기만 써야 할 것 같다는 생각이 들더라구요 ㅋㅋㅋㅋㅋㅋ
간혹 "이모지"가 아니라 "에모지"라고 쓰는 이유에 대한 질문을 받습니다. 여기다 써 두면 앞으로 링크만 던지면 되겠지?
요약: 에모지라서 에모지라고 씁니다.
"이모지"라는 표기는 아마도 "emoji"가 "emotion"이나 "emoticon"과 관련이 있다고 생각해서 나오는 것으로 보이는데요. "emoji"와 "emoticon"은 가짜동족어(false cognate)입니다. "emoji"는 일본어 絵文字(에모지)를 영어에서 그대로 받아들여 쓰고 있는 것입니다. 심지어 구성원리도 에모+지가 아니고 에+모지(絵+文字)입니다. "emotion"과 유사해 보이는 것은 순전히 우연일 뿐, 계통적으로 전혀 아무 상관이 없습니다. "이모티콘"과 "이미지"의 합성어가 아닙니다. (그랬으면 "-ji"가 아니라 "-ge"였겠죠.)
그리고 그렇기 때문에 에모지를 에모지로 표기할 실익이 생깁니다. :)
, ¯\_(ツ)_/¯
, ^_^
등은 이모티콘입니다. 반면 😂는 명확히 에모지입니다.
프로그래머에게 이건 정말 중요한 구분입니다. "이모티콘을 잘 표현하는 시스템"과 "에모지를 잘 표현하는 시스템"은 전혀 다른 과제이기 때문입니다. 에모지는 "그림 문자"라는 원래 뜻 그대로, 어떤 문자 집합(예를 들어 유니코드)에서 그림 문자가 "따로 있는" 것입니다. 내부 표현이야 어떻든, 적어도 최종 렌더링에서는 별도의 글리프가 할당되는 것이 에모지입니다. "무엇이 에모지이고 무엇이 에모지가 아닌가"는 상대적으로 명확합니다(문자 집합에 규정되어 있으니까).
반면 이모티콘은 "무엇이 이모티콘인가?"부터 불명확합니다. 우선 대부분의 이모티콘은 이모티콘이 아닌 문자를 조합하여 이모티콘이 만들어지는 형식입니다. 예를 들어 쌍점(:
)이나 닫는 괄호()
)는 그 자체로는 이모티콘이 아니지만 합쳐 놓으면 :)
이모티콘이 됩니다. 하지만 조합에 새로운 의미를 부여했다고 해서 다 이모티콘이라고 부르지도 않습니다. -_-
같은 것은 대다수가 이모티콘으로 인정하지만, ->
같은 것은 이모티콘이라고 부르지 않는 경향이 있습니다.
-
문자와 >
문자에는 화살표라는 의미가 없기 때문에, ->
조합과 화살표의 시각적 유사성에 기대어 화살표라는 새로운 의미로 "오용"한 것은 이모티콘의 구성 원리에 해당합니다. 하지만 화살표는 인간의 특정한 정서(emotion)에 대응하지 않으므로 이모티콘이라고는 잘 부르지 않습니다. 그렇다고 얼굴 표정을 나타내야만 이모티콘인가 하면 그렇지도 않습니다. orz
같은 것은 이모티콘으로 간주하는 경향이 있어 보입니다. 오징어를 나타내는 <:=
는 이모티콘인가? 이모티콘이 맞다면, 왜 ->
는 이모티콘이 아니고 <:=
는 이모티콘인가? 알 수 없습니다. ㅋㅋ
과 ㅠㅠ
는 둘 다 정서를 나타내는데, ㅠㅠ
만이 아이콘적 성질을 가지므로 이모티콘이고 ㅋ
는 이모티콘이 아닌가? 알 수 없습니다. 만약 ㅋ
만 이모티콘이 아니라고 한다면, ㅋ큐ㅠ
에서 큐
는 이모티콘인가 아닌가?? 알 수 없습니다. 이 알 수 없음은 이모티콘의 생래적 성질입니다. 어쩔 수 없죠.
JavaScript에 Object.map()
같은 함수 하나 있었으면 좋겠다. 맨날 Object.fromEntries(Object.entries(obj).map(...))
하기 귀찮다. (게다가 TypeScript에서 타입도 보존이 안 되어서 as
키워드 써야 함…)
@bglbgl gwyng
@xiniha 그러고 보니 최근 Node.js에서는
require(ESM)
이 된다고 들었던 것 같기도 한데, 그거랑은 상관 없는 얘기려나요?
@hongminhee洪 民憙 (Hong Minhee)
@bglbgl gwyng
require(ESM)
은 CJS에서 ESM을 갖다 쓰는 상황을 위한 거라서 이런 경우에 도움이 되지는 않습니다 🥲 ESM에서 CJS를 가져다 쓰려면 default import로 export 객체 전체를 import해서 쓰거나, createRequire()
로 require
함수를 직접 만들어서 써야 하는데, 둘 다 영 편한 방법은 아닙니다 😇
@bglbgl gwyng 헉… 어떻게 2025년에 ESM을 지원 안 하는 라이브러리가 그렇게 널리 쓰이고 있을 수 있는 걸까요…
@hongminhee洪 民憙 (Hong Minhee)
@bglbgl gwyng 리액트/릴레이도 CJS 빌드밖에 없습니다 ㅋㅋㅋㅋㅋ
Gemini Pro 2.5 엄청 똑똑하다... 기존 모델들은 대화가 길어지고 내용이 깊어지면 그냥 뻔한소리를 반복하기 시작하던데, 얘는 계속 생각해서 아이디어를 살려준다.
러스트에서 SIMD 코드 짜기 너무 고통스럽다 어떻게 좀 해달라... Rust 2024에선 unsafe_op_in_unsafe_fn
때문에 더 고통스러워졌다...
액펍 특성상 완전한 삭제는 매우 어렵습니다 (삭제 액티비티가 전달되지 않는 서버가 있을 수 있음)
일본에 Fediverse Linux Users Group이라는 모임이 있는데, 거기서 〈국한문혼용체에서 Hollo까지〉라는 이상한 주제로 오늘 발표를 합니다…
RE: https://hollo.social/@hongminhee/019603c7-b5ef-7d81-bbd4-4c37d46edce9
생각난 김에 또 하나 들자면, 본문에 대한 내부 표현이 HTML이 아니라 MFM이라는 것. MFM에 별 쓸 데 없는 기능은 다 넣어놓고, 정작 헤딩이나 목록 같이 문서의 의미론을 살리는 요소들은 HTML → MFM으로 번역을 안 해서 다 날아가 버린다. 서식에 굉장히 인색한 Mastodon조차도 원격에서 들어오는 HTML에 대해서는 Misskey보다 훨씬 많은 요소들을 받아들이는데 말이다.
가끔 보면 Misskey는 ActivityPub을 마지못해 지원하는 것 같다니까요. (실제로 만든 사람이 그런 뉘앙스의 얘기도 몇 번 했었고.)
Hackers' Pub의 소스 코드 저장소를 제 개인 계정에서 hackers-pub 조직 계정으로 옮겼습니다.
Hackers' Pub에 이제 알림 기능이 생겼습니다. 우상단 프로필 사진 바로 왼쪽에 알림 아이콘이 추가되었고, 이제 읽지 않은 알림이 있을 경우 그 위에 빨간 동그라미가 표시됩니다. 알림의 종류는 현재 다음 다섯 가지입니다:
- 누가 날 팔로했을 때
- 누가 날 언급했을 때
- 누가 내 글에 답글을 달았을 때
- 누가 내 글을 인용했을 때
- 누가 내 글을 공유했을 때
지금 Hackers' Pub에는 Fresh 2.0 알파를 쓰고 있는데, 여러 가지 아쉬운 점이 많지만 그 중 하나가 아일랜드 컴포넌트로 콘텍스트가 전달이 안 된다는 것. 현재 아일랜드 컴포넌트에는 로캘 같은 정보를 일일히 프랍(prop)으로 넘겨줘야 한다…
RE: https://hackers.pub/@kodingwarrior/0195f94d-61f3-7cab-8a34-f2a8ab0f9a4a
XiNiHa shared the below article:
셸 언어는 때로 추하길 요구 받는다
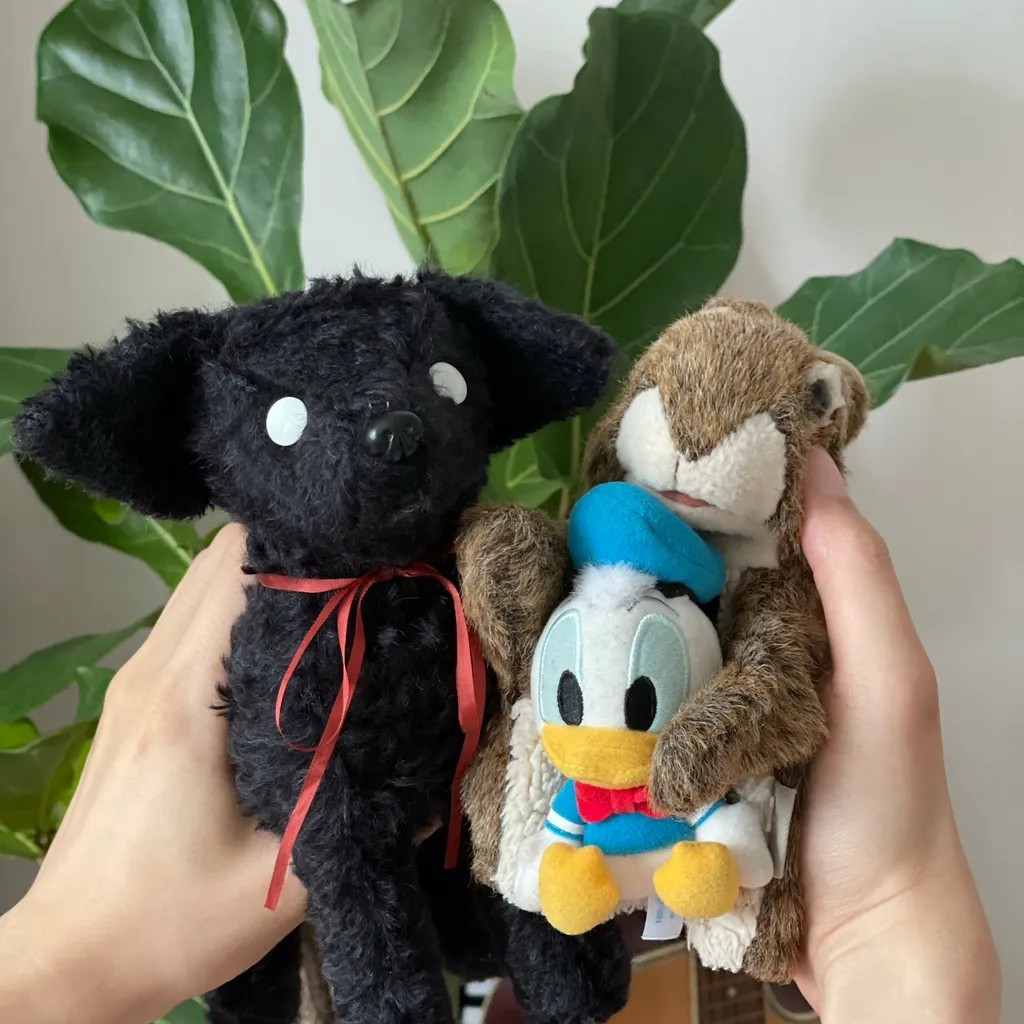
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
이 글에서는 명령줄 인터페이스(CLI)를 지배하는 셸 언어의 독특한 설계 철학을 탐구하며, 셸 언어가 왜 때로는 "추함"을 받아들여야 하는지에 대한 이유를 설명합니다. Bash와 PowerShell을 비교하며, PowerShell이 가독성을 높이기 위해 장황해진 반면, Bash는 간결함을 유지하여 빠른 상호작용에 더 적합함을 지적합니다. 현대적인 셸인 Nushell이 이 균형을 맞추기 위해 노력하는 점을 언급하며, 셸 언어의 성공은 "아름다운 코드"와 "효율적인 상호작용" 사이의 균형에 달려 있음을 강조합니다. 마지막으로, 모든 도구는 사용 맥락에 맞게 설계되어야 한다는 더 넓은 소프트웨어 설계 원칙을 제시하며, 셸 언어의 맥락은 키보드와 사용자 사이의 빠른 대화임을 강조합니다. 이 글은 셸 언어 설계에 대한 흥미로운 통찰력을 제공하며, 소프트웨어 설계 시 맥락의 중요성을 일깨워 줍니다.
Read more →사실 Hackers' Pub은 저희 집 홈 서버인 Mac mini M4 깡통 모델에서 돌아가고 있을 뿐만 아니라, 배포도 compose.yaml 파일의 image:
필드를 매번 손으로 고친 뒤 docker compose up -d
를 치는 전근대적인 방식으로 이뤄지고 있습니다… 뭔가 자동화를 하고 싶긴 한데 귀찮은 마음이 커서 아직까지 이대로 살고 있네요.
XiNiHa shared the below article:
deno-task-hooks: Git 훅을 Deno 태스크로 쉽게 관리하기
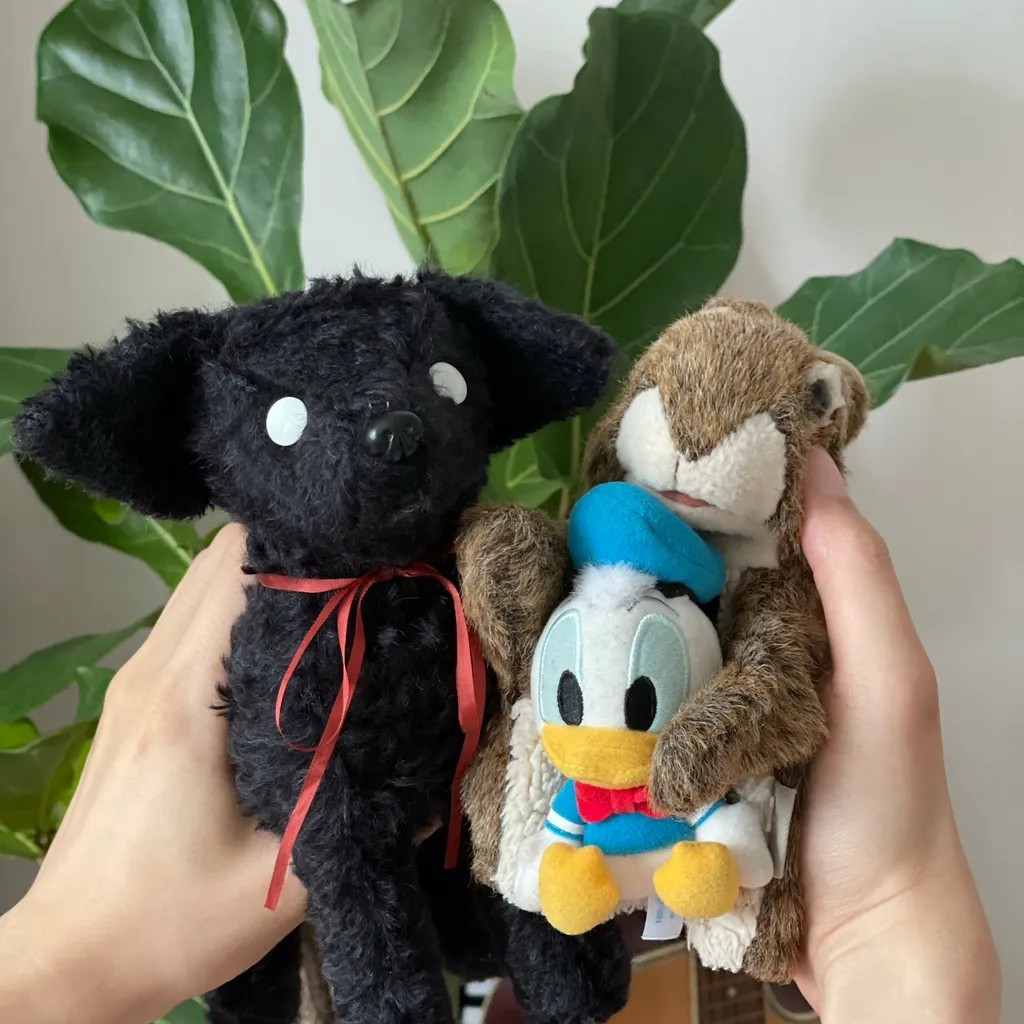
洪 民憙 (Hong Minhee) @hongminhee@hackers.pub
안녕하세요! 오늘은 제가 개발한 deno-task-hooks 패키지를 소개해 드리려고 합니다. 이 도구는 Deno 태스크를 Git 훅으로 사용할 수 있게 해주는 간단하면서도 유용한 패키지입니다.
어떤 문제를 해결하나요?
Git을 사용하는 개발 팀에서는 코드 품질 유지를 위해 커밋이나 푸시 전에 린트, 테스트 등의 검증 작업을 실행하는 것이 일반적입니다. 이러한 작업은 Git 훅을 통해 자동화할 수 있지만, 기존 방식에는 몇 가지 문제가 있었습니다:
- Git 훅 스크립트를 팀원들과 공유하기 어려움 (.git 디렉토리는 보통 버전 관리에서 제외됨)
- 각 개발자가 로컬에서 훅을 직접 설정해야 하는 번거로움
- 훅 스크립트의 일관성 유지가 어려움
deno-task-hooks는 이러한 문제를 해결하기 위해 Deno의 태스크 러너를 활용합니다. Deno 태스크는 deno.json 파일에 정의되어 버전 관리가 가능하므로, 팀 전체가 동일한 Git 훅을 쉽게 공유할 수 있습니다.
어떻게 작동하나요?
deno-task-hooks의 작동 방식은 간단합니다:
- deno.json 파일에 Git 훅으로 사용할 Deno 태스크를 정의합니다.
hooks:install
태스크를 실행하면, 정의된 태스크들이 자동으로 .git/hooks/ 디렉토리에 설치됩니다.- 이후 Git 작업 시 해당 훅이 트리거되면 연결된 Deno 태스크가 실행됩니다.
설치 및 사용 방법
1. hooks:install 태스크 추가하기
먼저 deno.json 파일에 hooks:install
태스크를 추가합니다:
{
"tasks": {
"hooks:install": "deno run --allow-read=deno.json,.git/hooks/ --allow-write=.git/hooks/ jsr:@hongminhee/deno-task-hooks"
}
}
2. Git 훅 정의하기
Git 훅은 hooks:
접두사 다음에 훅 이름(케밥 케이스)을 붙여 정의합니다. 예를 들어, pre-commit
훅을 정의하려면:
{
"tasks": {
"hooks:pre-commit": "deno check *.ts && deno lint"
}
}
3. 훅 설치하기
다음 명령어를 실행하여 정의된 훅을 설치합니다:
deno task hooks:install
이제 Git 커밋을 실행할 때마다 pre-commit
훅이 자동으로 실행되어 TypeScript 파일을 검사하고 린트 검사를 수행합니다.
지원되는 Git 훅 종류
deno-task-hooks는 다음과 같은 모든 Git 훅 타입을 지원합니다:
applypatch-msg
commit-msg
fsmonitor-watchman
post-update
pre-applypatch
pre-commit
pre-merge-commit
pre-push
pre-rebase
pre-receive
prepare-commit-msg
push-to-checkout
sendemail-validate
update
이점
deno-task-hooks를 사용하면 다음과 같은 이점이 있습니다:
- 간편한 공유: Git 훅을 deno.json 파일에 정의하여 팀 전체가 동일한 훅을 사용할 수 있습니다.
- 설정 용이성: 새 팀원은 저장소를 클론한 후 한 번의 명령어로 모든 훅을 설치할 수 있습니다.
- 유지 관리 용이성: 훅 스크립트를 중앙에서 관리하므로 변경 사항을 쉽게 추적하고 적용할 수 있습니다.
- Deno의 안전성: Deno의 권한 모델을 활용하여 훅 스크립트의 보안을 강화할 수 있습니다.
마치며
deno-task-hooks는 작은 패키지이지만, Git과 Deno를 함께 사용하는 팀의 개발 경험을 크게 향상시킬 수 있습니다. 코드 품질 유지와 개발 워크플로우 자동화를 위해 한번 사용해 보세요!
패키지는 JSR에서 다운로드할 수 있으며, GitHub에서 소스 코드를 확인할 수 있습니다.
피드백과 기여는 언제나 환영합니다! 😊